循环双链表 值排序 c语言
时间: 2024-02-15 14:29:17 浏览: 17
以下是循环双链表的值排序的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
struct Node* prev;
};
void insertAtEnd(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
struct Node* last = (*head_ref);
new_node->data = new_data;
new_node->next = (*head_ref);
if ((*head_ref) == NULL) {
new_node->prev = new_node;
(*head_ref) = new_node;
return;
}
while (last->next != (*head_ref))
last = last->next;
last->next = new_node;
new_node->prev = last;
(*head_ref)->prev = new_node;
}
void bubbleSort(struct Node** head_ref) {
struct Node *i, *j;
int temp;
for (i = (*head_ref); i->next != (*head_ref); i = i->next) {
for (j = i->next; j != (*head_ref); j = j->next) {
if (i->data > j->data) {
temp = i->data;
i->data = j->data;
j->data = temp;
}
}
}
}
void printList(struct Node* node) {
struct Node* temp = node;
if (node != NULL) {
do {
printf("%d ", temp->data);
temp = temp->next;
} while (temp != node);
}
}
int main() {
struct Node* head = NULL;
insertAtEnd(&head, 4);
insertAtEnd(&head, 2);
insertAtEnd(&head, 1);
insertAtEnd(&head, 3);
printf("Original List: ");
printList(head);
bubbleSort(&head);
printf("\nSorted List: ");
printList(head);
return 0;
}
```
该代码使用冒泡排序算法对循环双链表进行排序。在每次迭代中,它比较相邻的节点,并交换它们的位置,以便将最小的节点移到列表的前面。最终,整个列表将按升序排列。
注意,这里的循环双链表采用头尾相连的方式,即最后一个节点的 next 指向头节点,头节点的 prev 指向最后一个节点。这样,我们就可以遍历整个列表,同时也可以很方便地将新节点插入到列表的末尾。
相关推荐
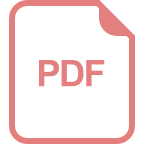














