在linux下使用c语言创建线程
时间: 2023-03-03 15:49:49 浏览: 136
在 Linux 下,可以使用 C 语言中的 `pthread` 库来创建和管理线程。下面是一个简单的示例程序,演示了如何使用 `pthread` 库创建一个线程。
```c
#include <pthread.h>
#include <stdio.h>
void* thread_function(void* arg)
{
printf("Hello from the thread!\n");
pthread_exit(NULL);
}
int main()
{
pthread_t my_thread;
int ret = pthread_create(&my_thread, NULL, thread_function, NULL);
if (ret != 0) {
printf("Failed to create thread.\n");
return 1;
}
printf("Hello from the main program.\n");
pthread_join(my_thread, NULL);
return 0;
}
```
这个程序创建了一个名为 `my_thread` 的新线程,并将其绑定到 `thread_function` 函数。在 `main` 函数中,它打印一条消息,然后等待线程执行完成。当 `my_thread` 线程执行时,它将打印另一条消息。注意,在这个示例程序中,我们使用了 `pthread_join` 函数等待线程的执行完成。如果不等待线程完成就退出程序,那么线程的执行可能会被中断。
要编译这个程序,您需要使用以下命令:
```
gcc -pthread -o myprogram myprogram.c
```
其中 `-pthread` 选项指示编译器链接 `pthread` 库。执行程序 `myprogram` 将输出以下内容:
```
Hello from the main program.
Hello from the thread!
```
这表明程序中的线程已经成功创建并在后台执行。
阅读全文
相关推荐
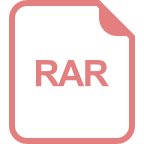
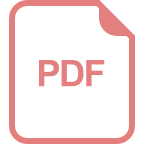
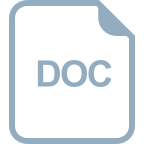
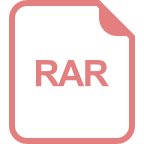
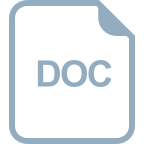
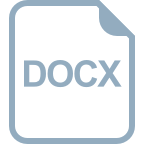
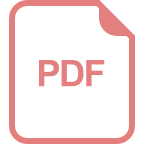
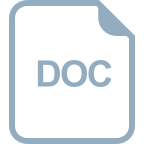
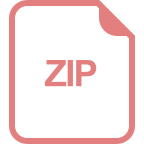
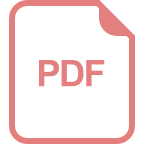
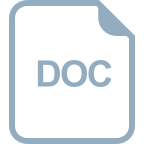
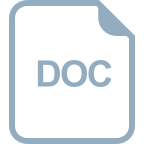
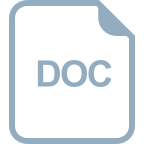
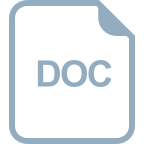
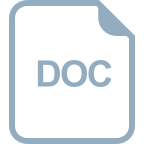