Write pytorch-based Python code to implement a neural network that solves binary classification problem with an output layer of 3 weighted sub-networks. The first weight is Positive, the second is negative, which is random and take the opposite of the absolute value of the standard normal distribution, the third random weight obeys a standard normal distribution. The exact value of the 3 weights are obtained from the objective function by Back propagation. The activation function is set to tanh.
时间: 2024-04-08 17:30:16 浏览: 100
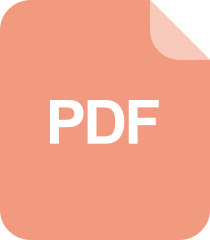
Pytorch实现神经网络的分类方式
Certainly! Here's an example of PyTorch-based Python code that implements a neural network for binary classification with an output layer of 3 weighted sub-networks using the tanh activation function:
```python
import torch
import torch.nn as nn
import torch.optim as optim
class NeuralNetwork(nn.Module):
def __init__(self, input_size):
super(NeuralNetwork, self).__init__()
self.fc1 = nn.Linear(input_size, 10)
self.fc2 = nn.Linear(10, 10)
self.fc3 = nn.Linear(10, 3)
def forward(self, x):
x = torch.tanh(self.fc1(x))
x = torch.tanh(self.fc2(x))
x = self.fc3(x)
return x
# Generate random weights for the second sub-network
negative_weights = -torch.abs(torch.randn(10))
# Create an instance of the neural network
input_size = 10 # Replace with the actual input size
model = NeuralNetwork(input_size)
# Define the loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.1)
# Training loop
for epoch in range(100):
# Forward pass
outputs = model(inputs) # Replace inputs with your actual input data
loss = criterion(outputs, labels) # Replace labels with your actual labels
# Backward and optimize
optimizer.zero_grad()
loss.backward()
optimizer.step()
# Update the second sub-network's weight using backpropagation
model.fc2.weight.data = negative_weights
# Print the loss during training
print(f"Epoch {epoch+1}/{num_epochs}, Loss: {loss.item():.4f}")
```
In this code, we define a `NeuralNetwork` class that inherits from `nn.Module` and implements the forward pass. The network consists of three fully connected layers (`nn.Linear`) with tanh activation for the hidden layers. The second sub-network's weight is initialized with random negative values obtained from the standard normal distribution.
During the training loop, we compute the forward pass, calculate the loss with the `nn.CrossEntropyLoss` function, perform backpropagation, and update the weights using the optimizer (`optim.SGD`). The second sub-network's weight is updated after each backpropagation step.
Please replace `input_size`, `inputs`, and `labels` with your actual input size, input data, and labels, respectively. Adjust the hyperparameters and the number of training epochs according to your specific problem.
Note: This code is a basic example and may need modifications to fit your specific use case.
阅读全文
相关推荐
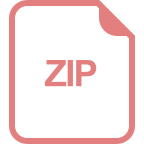

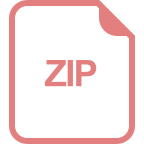
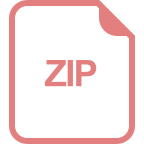
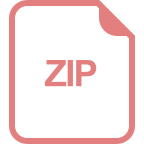
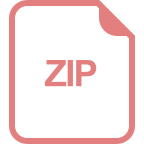
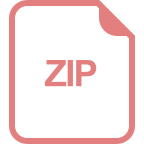
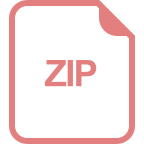
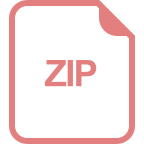
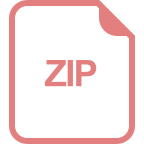
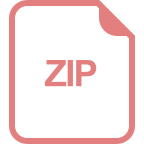
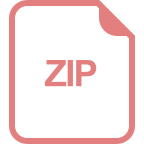