写一段预测房价的Python代码
时间: 2023-03-13 12:16:52 浏览: 99
# 导入必要的库
import numpy as np
import pandas as pd
from sklearn.linear_model import LinearRegression# 从csv文件中读取数据
data = pd.read_csv('house_data.csv')# 分离特征和标签
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values# 建立线性回归模型
model = LinearRegression()
model.fit(X, y)# 对房价进行预测
house_price = model.predict([[2000, 2, 1, 2]])
print("预测房价为:", house_price[0])
相关问题
写一段预测房价的python代码
以下是一段简单的使用线性回归算法预测房价的Python代码示例:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
# 假设我们已经有了训练数据 X_train 和对应的房价标签 y_train
X_train = np.array([[1500], [2000], [2500], [3000], [3500]])
y_train = np.array([120000, 150000, 180000, 210000, 240000])
# 创建线性回归模型
model = LinearRegression()
# 训练模型
model.fit(X_train, y_train)
# 假设我们要预测一套房子的价格,它的面积为 2800 平方英尺
X_test = np.array([[2800]])
# 预测房价
y_pred = model.predict(X_test)
# 输出预测结果
print("预测房价为:$%.2f" % y_pred)
```
代码解释:
1. 首先导入必要的库,包括 numpy 和 scikit-learn 中的 LinearRegression 模型。
2. 假设我们已经有了训练数据 X_train 和对应的房价标签 y_train,其中 X_train 代表房子的面积,y_train 代表对应的房价。
3. 创建 LinearRegression 模型,并使用 fit() 方法训练模型。
4. 假设我们要预测一套房子的价格,它的面积为 2800 平方英尺,将其存储在 X_test 中。
5. 使用 predict() 方法预测房价。
6. 输出预测结果,其中格式化字符串使用了“$%.2f”来将预测结果格式化为美元金额,保留两位小数。
帮我写一段波士顿房价预测的Python代码
好的,以下是一个简单的波士顿房价预测的Python代码,使用的是线性回归模型:
```python
# 导入必要的库和数据集
from sklearn.datasets import load_boston
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
# 加载数据集
boston = load_boston()
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(boston.data, boston.target, test_size=0.2, random_state=42)
# 创建线性回归模型
model = LinearRegression()
# 用训练集训练模型
model.fit(X_train, y_train)
# 用测试集评估模型
score = model.score(X_test, y_test)
print("模型得分:", score)
# 预测房价
new_data = [[0.2, 0, 6.0, 0, 0.5, 6.5, 65.2, 4.09, 1, 296, 15.3, 396.9, 4.98]]
prediction = model.predict(new_data)
print("预测房价:", prediction)
```
注:以上代码仅供参考,实际应用中还需要进行数据预处理、特征工程等步骤。
阅读全文
相关推荐
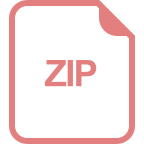









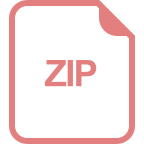
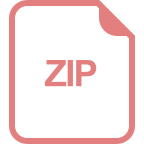


