join() argument must be str or bytes, not 'list'
时间: 2024-01-28 21:03:45 浏览: 38
This error occurs when you try to use the `join()` method with a list as an argument. The `join()` method can only be used with strings or bytes, not with a list.
To fix this error, you need to convert the list to a string or byte object before using the `join()` method. For example:
```
my_list = ['a', 'b', 'c']
my_string = ''.join(my_list)
print(my_string)
```
Output:
```
'abc'
```
In this example, we first create a list `my_list` with three elements. We then convert the list to a string using the `join()` method and store the result in a variable `my_string`. Finally, we print the value of `my_string` which is `'abc'`.
相关问题
join() argument must be str, bytes, or os.PathLike object, not 'list'
这个错误通常是由于将一个列表作为参数传递给了`join()`方法,而`join()`方法的参数应该是字符串、字节或路径对象。为了解决这个问题,你需要将列表转换为字符串或是将列表中的元素拼接成字符串,然后再将其传递给`join()`方法。
以下是一个示例代码,将列表转换为字符串:
```
my_list = ['apple', 'banana', 'orange']
my_str = ','.join(my_list)
```
在这个示例中,`my_list`是一个包含三个元素的列表,`my_str`是将这三个元素用逗号连接起来的字符串。
如果你想将列表中的元素拼接成一个字符串,可以使用列表推导式:
```
my_list = ['apple', 'banana', 'orange']
my_str = ''.join([str(elem) for elem in my_list])
```
这将把`my_list`中的元素转换为字符串并将它们拼接在一起。
TypeError: join() argument must be str, bytes, or os.PathLike object, not 'list'
This error occurs when you try to use the join() method with a list as an argument instead of a string, bytes, or a PathLike object. The join() method is used to concatenate a list of strings into a single string with a delimiter.
To fix this error, you need to convert the list to a string or a PathLike object before passing it to the join() method. Here's an example:
```
my_list = ['apple', 'banana', 'orange']
delimiter = ', '
# Convert the list to a string before joining
my_string = delimiter.join(my_list)
print(my_string)
# Output: 'apple, banana, orange'
```
In this example, we first define a list called `my_list` and a delimiter called `delimiter`. We then convert the list to a string using the join() method and store the result in the `my_string` variable. Finally, we print the `my_string` variable, which outputs the concatenated string with the delimiter.
相关推荐
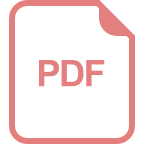
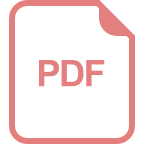
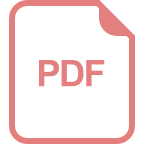





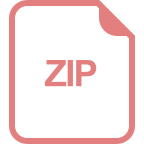
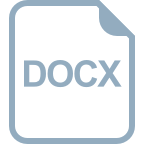
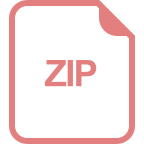