C++ QToolButton点击后出现在QtDraawingPaperEditor中出现可以随着鼠标移动的图片,点击左键后,图片被放下
时间: 2024-05-08 15:20:28 浏览: 12
您可以通过以下步骤实现:
1. 创建一个QGraphicsScene对象和一个QGraphicsPixmapItem对象。将QGraphicsPixmapItem对象添加到QGraphicsScene对象中。
2. 在QToolButton的clicked()信号中,显示一个QFileDialog来选择要放置的图像文件。将所选文件加载到QPixmap对象中。
3. 将QPixmap对象设置为QGraphicsPixmapItem对象的pixmap,并根据需要调整其大小。
4. 在QGraphicsScene对象中添加该QGraphicsPixmapItem对象。
5. 在QGraphicsScene对象上设置一个捕捉鼠标事件的事件过滤器。
6. 在事件过滤器中,捕捉鼠标按下和释放事件。在鼠标按下时,将QGraphicsPixmapItem对象从QGraphicsScene对象中移除,并将其添加到QGraphicsView对象中。然后,在QGraphicsView对象上设置一个捕捉鼠标事件的事件过滤器。
7. 在QGraphicsView对象的事件过滤器中,捕捉鼠标释放事件。在鼠标释放时,将QGraphicsPixmapItem对象移动到QGraphicsScene对象中,并将其位置设置为鼠标释放的位置。
下面是一个示例代码,演示如何实现上述步骤:
```cpp
#include <QtWidgets>
class DrawingPaperEditor : public QGraphicsView
{
public:
DrawingPaperEditor(QWidget *parent = nullptr);
protected:
bool eventFilter(QObject *obj, QEvent *event) override;
private:
QGraphicsScene *m_scene;
QGraphicsPixmapItem *m_pixmapItem;
QPixmap m_pixmap;
};
DrawingPaperEditor::DrawingPaperEditor(QWidget *parent)
: QGraphicsView(parent)
, m_scene(new QGraphicsScene(this))
, m_pixmapItem(new QGraphicsPixmapItem)
{
setScene(m_scene);
m_scene->addItem(m_pixmapItem);
// Install event filter on the scene to capture mouse press events.
m_scene->installEventFilter(this);
}
bool DrawingPaperEditor::eventFilter(QObject *obj, QEvent *event)
{
if (obj == m_scene) {
if (event->type() == QEvent::GraphicsSceneMousePress) {
QMouseEvent *mouseEvent = static_cast<QMouseEvent *>(event);
if (mouseEvent->button() == Qt::LeftButton) {
// Remove pixmap item from scene and add it to view.
m_scene->removeItem(m_pixmapItem);
QGraphicsView *view = qobject_cast<QGraphicsView *>(parent());
if (view) {
view->scene()->addItem(m_pixmapItem);
view->installEventFilter(this);
}
}
} else if (event->type() == QEvent::GraphicsSceneMouseRelease) {
QMouseEvent *mouseEvent = static_cast<QMouseEvent *>(event);
if (mouseEvent->button() == Qt::LeftButton) {
// Move pixmap item to scene and set its position to mouse release position.
QGraphicsView *view = qobject_cast<QGraphicsView *>(parent());
if (view) {
QPointF pos = view->mapToScene(mouseEvent->pos());
m_pixmapItem->setPos(pos);
view->scene()->removeItem(m_pixmapItem);
m_scene->addItem(m_pixmapItem);
view->removeEventFilter(this);
}
}
}
} else if (obj == parent()) {
if (event->type() == QEvent::MouseButtonRelease) {
QGraphicsView *view = qobject_cast<QGraphicsView *>(parent());
if (view) {
QPointF pos = view->mapToScene(static_cast<QMouseEvent *>(event)->pos());
m_pixmapItem->setPos(pos);
}
}
}
return false;
}
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QToolButton toolButton;
toolButton.setText("Add Image");
DrawingPaperEditor editor;
QVBoxLayout layout;
layout.addWidget(&toolButton);
layout.addWidget(&editor);
QWidget widget;
widget.setLayout(&layout);
widget.show();
QObject::connect(&toolButton, &QToolButton::clicked, [&](){
QString fileName = QFileDialog::getOpenFileName(&widget, "Open Image", QString(), "Images (*.png *.xpm *.jpg)");
if (!fileName.isEmpty()) {
QPixmap pixmap(fileName);
if (!pixmap.isNull()) {
editor.setSceneRect(pixmap.rect());
editor.m_pixmap = pixmap;
editor.m_pixmapItem->setPixmap(pixmap.scaled(editor.width(), editor.height(), Qt::KeepAspectRatio));
editor.m_pixmapItem->setPos(editor.sceneRect().center());
}
}
});
return a.exec();
}
```
相关推荐
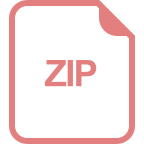
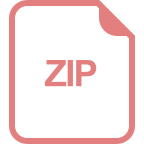














