写一个程序,用网络数据和crf实现中文分词
时间: 2024-05-26 11:17:38 浏览: 161
这是一个比较复杂的任务,需要涉及到自然语言处理、神经网络和条件随机场等知识。下面是一个简单的程序框架,供参考:
1. 数据预处理
首先需要准备训练数据,包括中文文本和标注好的分词结果。可以使用公开的中文分词数据集,比如SIGHAN,或者自己手动标注。然后需要将数据转换成模型需要的格式,比如将中文字符转换成向量表示。
2. 神经网络训练
使用深度学习框架,比如TensorFlow或PyTorch,搭建一个中文分词的神经网络模型,比如基于LSTM或Transformer的模型。然后使用训练数据对模型进行训练,调整模型参数,使得模型能够准确地预测中文分词结果。
3. 条件随机场模型
在神经网络输出中,每个字符都有一个概率分布,表示该字符属于哪个词。但是单独考虑每个字符的概率分布,并不能保证分词结果的连续性。因此需要采用条件随机场(CRF)模型,对神经网络的输出进行优化。CRF是一种序列标注模型,可以考虑相邻字符之间的关系,从而得到更优的分词结果。
4. 模型评估
使用测试数据对模型进行评估,比如计算准确率、召回率和F1值等指标,以确定模型的性能。
5. 应用部署
最后将训练好的模型部署到应用中,接收输入的中文文本,返回分词结果。
以上是一个大致的程序框架,具体实现还需要根据实际情况进行调整。
相关问题
写一个程序,用crf实现中文分词
好的,下面是一个简单的中文分词程序,使用CRF模型实现:
```python
import pycrfsuite
import jieba
# 读取训练数据
def read_data(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
data = f.readlines()
return [line.strip().split() for line in data]
# 特征提取函数
def extract_features(sentence):
features = []
for i in range(len(sentence)):
features.append([
'BOS' if i == 0 else 'NOT_BOS',
'EOS' if i == len(sentence) - 1 else 'NOT_EOS',
'SINGLE' if len(sentence[i]) == 1 else 'NOT_SINGLE',
'FIRST_' + sentence[i][0],
'LAST_' + sentence[i][-1],
'PREFIX_' + sentence[i][:2],
'SUFFIX_' + sentence[i][-2:],
'IN_DICT' if sentence[i] in word_dict else 'NOT_IN_DICT'
])
return features
# 训练模型
def train_model(train_data, model_path):
trainer = pycrfsuite.Trainer(verbose=False)
for sentence, labels in train_data:
features = extract_features(sentence)
trainer.append(features, labels)
trainer.set_params({
'c1': 1.0,
'c2': 1e-3,
'max_iterations': 100,
'feature.possible_transitions': True
})
trainer.train(model_path)
# 加载字典
def load_dict(dict_path):
with open(dict_path, 'r', encoding='utf-8') as f:
word_dict = set([line.strip() for line in f.readlines()])
return word_dict
# 分词函数
def segment(sentence, model_path, word_dict):
model = pycrfsuite.Tagger()
model.open(model_path)
features = extract_features(sentence)
labels = model.tag(features)
segment_list = []
for i in range(len(labels)):
if labels[i] == 'B' or labels[i] == 'M':
segment_list[-1] += sentence[i]
else:
segment_list.append(sentence[i])
# 使用jieba对未分出的词进行分词
result = []
for segment in segment_list:
if segment in word_dict:
result.append(segment)
else:
result += jieba.lcut(segment)
return result
if __name__ == '__main__':
train_data = read_data('train.txt')
word_dict = load_dict('dict.txt')
train_model(train_data, 'model.crfsuite')
sentence = '今天天气真好,适合出去玩。'
print(segment(sentence, 'model.crfsuite', word_dict))
```
其中,`train.txt`是训练数据,每行是一个句子和对应的分词结果,用空格分隔;`dict.txt`是自定义的字典,用于判断词语是否在字典中出现过。在训练模型和分词时,我们使用了一些手工设计的特征,包括词首字、词尾字、前缀、后缀等。对于未能分出的词语,我们使用jieba库进行分词。
阅读全文
相关推荐
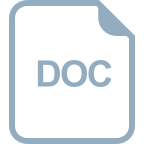
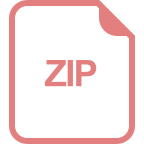
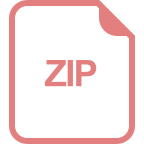
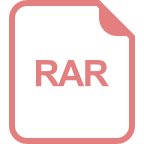
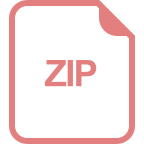
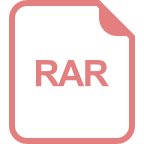
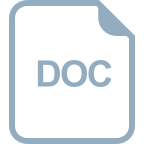
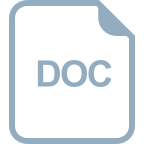
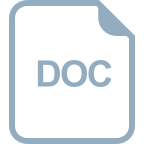
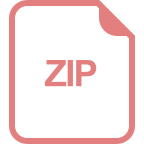
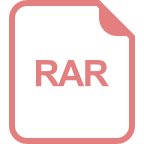
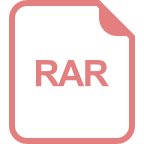
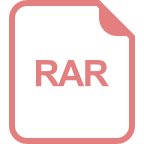
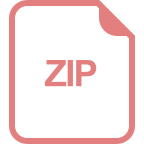
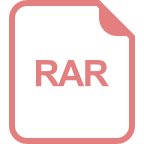