请解释以下代码<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" isErrorPage="true"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% exception.printStackTrace(response.getWriter()); %> </body> </html>
时间: 2023-07-02 16:09:22 浏览: 146
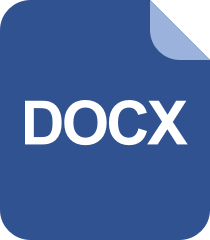
第六章 JSP技术.docx
这是一个 JSP(Java Server Pages)页面,用于显示发生异常时的错误信息。在 JSP 页面中,使用<% %>标记来执行 Java 代码。在这个例子中,使用了内置的异常对象exception和response.getWriter()来获取异常信息并将其输出到浏览器上。当 isErrorPage 属性设置为 true 时,这个 JSP 页面可以被用作错误页面,当服务器发生异常时,该页面会被自动调用。同时,该页面的 contentType 和 pageEncoding 属性设置为 UTF-8,确保页面以 UTF-8 编码显示。
阅读全文
相关推荐
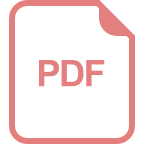
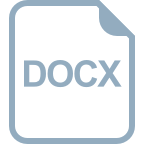


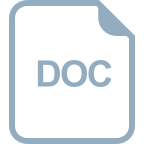
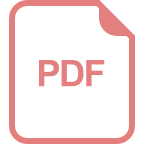
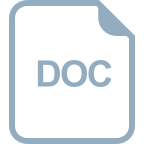
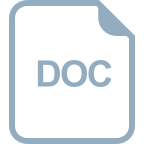
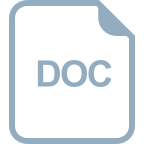
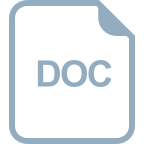
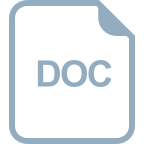
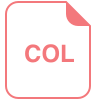
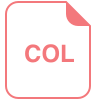

