为什么当我鼠标停在红色方块上,蓝色方块不会出现?
时间: 2024-04-08 10:31:12 浏览: 69
根据你提供的HTML代码片段,我看到一个问题。在CSS中,你使用了子选择器(.dt:hover .dd)来选择红色方块(class="dt")悬停时显示的蓝色方块(class="dd")。然而,这两个元素并不是父子关系,它们是同级元素。因此,你需要使用兄弟选择器(+)或者修改HTML结构来实现期望效果。
如果你想使用兄弟选择器,可以将HTML代码修改为以下形式:
```html
<div class="dt"></div>
<div class="dd"></div>
```
然后将CSS代码修改为:
```css
.dt:hover + .dd {
display: block;
}
```
这样,当鼠标悬停在红色方块上时,紧接着的下一个同级元素的蓝色方块会显示出来。
另外,如果你希望蓝色方块是红色方块的子元素,可以将HTML代码修改为以下形式:
```html
<div class="dt">
<div class="dd"></div>
</div>
```
然后保持CSS代码不变。这样,当鼠标悬停在红色方块上时,内部的蓝色方块会显示出来。
请根据你的需求选择适合的解决方案。
相关问题
请给出具体代码,使得点击后方块从蓝色变为绿色
为了实现在JavaScript的HTML5 Canvas上,当用户点击一个蓝色方块时将其变绿,首先需要创建一个蓝色方块,然后添加点击事件监听器。下面是一个简单的例子:
1. HTML结构:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Click to Change Block Color</title>
</head>
<body>
<canvas id="myCanvas" width="200" height="200"></canvas>
</body>
</html>
```
2. JavaScript代码:
```javascript
// 获取Canvas和2D上下文
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
// 定义一个蓝色方块的颜色和初始坐标
let blockColor = "blue";
let blockX = 50;
let blockY = 50;
let blockSize = 50;
// 绘制蓝色方块
function drawBlock() {
ctx.fillStyle = blockColor;
ctx.fillRect(blockX, blockY, blockSize, blockSize);
}
// 添加点击事件监听器
canvas.addEventListener("click", function(event) {
// 计算鼠标点击位置相对于Canvas的像素值
const mouseX = event.clientX - canvas.offsetLeft;
const mouseY = event.clientY - canvas.offsetTop;
// 检查点击位置是否在方块内
if (mouseX >= blockX && mouseX <= blockX + blockSize &&
mouseY >= blockY && mouseY <= blockY + blockSize) {
// 将蓝色改为绿色
blockColor = "green";
// 重新绘制方块
drawBlock();
}
});
// 初始化并绘制蓝色方块
drawBlock();
// 动画帧处理
function animate() {
requestAnimationFrame(animate);
}
animate();
```
在这个示例中,当你点击蓝色方块时,它的颜色会变为绿色。如果不在方块范围内,则不会变化。
使用python实现A*算法,要求可以使用鼠标点击方块选择起点、终点,障碍物是随机生成的
好的,我可以为您提供一个基本的 A*算法的实现,并添加鼠标交互和随机障碍物的功能。在代码中,我将使用 Pygame 库来处理鼠标事件和绘制方块。
首先,需要安装 Pygame 库。可以使用以下命令在终端中安装:
```
pip install pygame
```
然后,我们可以开始编写代码。下面是完整的代码:
```python
import pygame
from queue import PriorityQueue
import random
# 设置棋盘大小和方块大小
WIDTH = 800
WIN = pygame.display.set_mode((WIDTH, WIDTH))
pygame.display.set_caption("A* Path Finding Algorithm")
BLOCK_SIZE = 20
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
# 定义方块类
class Block:
def __init__(self, row, col):
self.row = row
self.col = col
self.x = row * BLOCK_SIZE
self.y = col * BLOCK_SIZE
self.color = WHITE
self.neighbors = []
def get_pos(self):
return self.row, self.col
def is_barrier(self):
return self.color == BLACK
def reset(self):
self.color = WHITE
def make_start(self):
self.color = GREEN
def make_barrier(self):
self.color = BLACK
def make_end(self):
self.color = RED
def make_path(self):
self.color = YELLOW
def draw(self):
pygame.draw.rect(WIN, self.color, (self.x, self.y, BLOCK_SIZE, BLOCK_SIZE))
# 添加邻居
def add_neighbors(self, grid):
if self.row < len(grid) - 1 and not grid[self.row + 1][self.col].is_barrier(): # 下
self.neighbors.append(grid[self.row + 1][self.col])
if self.row > 0 and not grid[self.row - 1][self.col].is_barrier(): # 上
self.neighbors.append(grid[self.row - 1][self.col])
if self.col < len(grid[0]) - 1 and not grid[self.row][self.col + 1].is_barrier(): # 右
self.neighbors.append(grid[self.row][self.col + 1])
if self.col > 0 and not grid[self.row][self.col - 1].is_barrier(): # 左
self.neighbors.append(grid[self.row][self.col - 1])
def __lt__(self, other):
return False
# 定义启发函数
def h(node1, node2):
return abs(node1.row - node2.row) + abs(node1.col - node2.col)
# 定义算法函数
def a_star_algorithm(grid, start, end):
count = 0
open_set = PriorityQueue()
open_set.put((0, count, start))
came_from = {}
g_score = {block: float("inf") for row in grid for block in row}
g_score[start] = 0
f_score = {block: float("inf") for row in grid for block in row}
f_score[start] = h(start, end)
open_set_hash = {start}
while not open_set.empty():
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
current = open_set.get()[2]
open_set_hash.remove(current)
if current == end:
reconstruct_path(came_from, end)
end.make_end()
return True
for neighbor in current.neighbors:
temp_g_score = g_score[current] + 1
if temp_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = temp_g_score
f_score[neighbor] = temp_g_score + h(neighbor, end)
if neighbor not in open_set_hash:
count += 1
open_set.put((f_score[neighbor], count, neighbor))
open_set_hash.add(neighbor)
neighbor.color = BLUE
draw_grid(grid)
if current != start:
current.color = YELLOW
end.color = RED
pygame.display.update()
if start in open_set_hash:
start.color = GREEN
else:
start.color = BLACK
return False
# 重构路径
def reconstruct_path(came_from, current):
while current in came_from:
current = came_from[current]
current.make_path()
# 绘制网格
def draw_grid(grid):
for row in grid:
for block in row:
block.draw()
for i in range(len(grid)):
pygame.draw.line(WIN, BLACK, (0, i * BLOCK_SIZE), (WIDTH, i * BLOCK_SIZE))
for j in range(len(grid[0])):
pygame.draw.line(WIN, BLACK, (j * BLOCK_SIZE, 0), (j * BLOCK_SIZE, WIDTH))
pygame.display.update()
# 创建随机障碍物
def create_barriers(grid):
for i in range(len(grid)):
for j in range(len(grid[0])):
if random.randint(0, 3) == 0:
grid[i][j].make_barrier()
# 初始化棋盘
def init_grid():
grid = []
for i in range(int(WIDTH / BLOCK_SIZE)):
row = []
for j in range(int(WIDTH / BLOCK_SIZE)):
block = Block(i, j)
row.append(block)
grid.append(row)
for i in range(len(grid)):
for j in range(len(grid[0])):
grid[i][j].add_neighbors(grid)
return grid
# 主函数
def main():
run = True
started = False
grid = init_grid()
start = None
end = None
while run:
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
if started:
continue
if pygame.mouse.get_pressed()[0]: # 鼠标左键按下
pos = pygame.mouse.get_pos()
row = pos[0] // BLOCK_SIZE
col = pos[1] // BLOCK_SIZE
block = grid[row][col]
if not start and block != end:
start = block
start.make_start()
elif not end and block != start:
end = block
end.make_end()
elif block != start and block != end:
block.make_barrier()
elif pygame.mouse.get_pressed()[2]: # 鼠标右键按下
pos = pygame.mouse.get_pos()
row = pos[0] // BLOCK_SIZE
col = pos[1] // BLOCK_SIZE
block = grid[row][col]
block.reset()
if block == start:
start = None
elif block == end:
end = None
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE and not started:
for row in grid:
for block in row:
block.add_neighbors(grid)
create_barriers(grid)
a_star_algorithm(grid, start, end)
pygame.quit()
if __name__ == "__main__":
main()
```
运行代码后,您将看到一个棋盘,您可以使用鼠标左键选择起点、终点,使用鼠标右键删除方块,然后按下空格键运行 A*算法。障碍物将自动随机分布在棋盘上。当算法运行时,您将看到蓝色方块表示算法正在搜索的路径,黄色方块表示搜索到的路径,红色方块表示终点,绿色方块表示起点。
希望这能帮助您开始实现 A*算法。
阅读全文
相关推荐
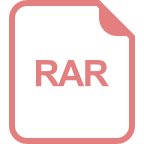
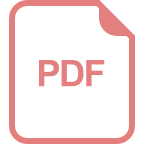
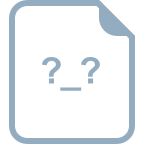
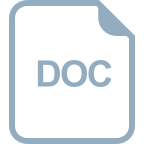
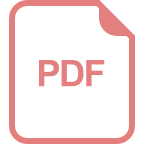
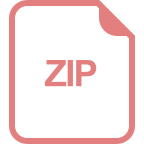
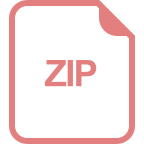
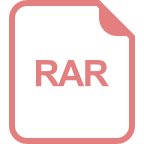
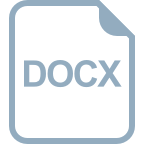
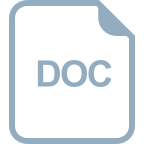
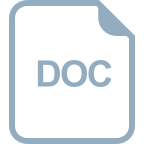
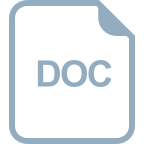



