python缩放图片并保存
时间: 2023-06-28 07:11:10 浏览: 108
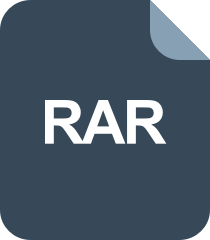
保存图片缩放
你可以使用 Python 的 Pillow 库来缩放图片并保存。以下是一个示例代码:
```python
from PIL import Image
# 打开图片
image = Image.open('example.jpg')
# 缩放图片
new_size = (image.width // 2, image.height // 2)
resized_image = image.resize(new_size)
# 保存图片
resized_image.save('example_resized.jpg')
```
在这个示例中,我们使用 `Image.open()` 打开了一张名为 `example.jpg` 的图片。然后,我们使用 `image.resize()` 方法将图片缩放到原来的一半大小,并将结果保存为 `example_resized.jpg`。
阅读全文
相关推荐
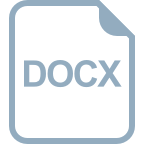





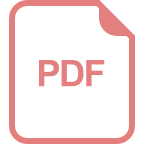
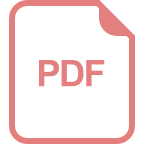
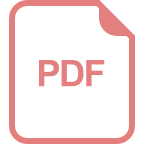





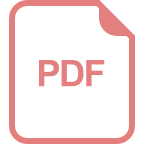