帮我提供一个使用python写的鲸鱼优化算法
时间: 2023-08-28 08:11:33 浏览: 92
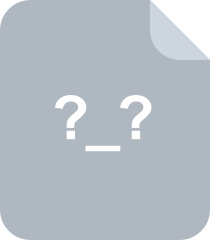
鲸鱼优化算法python代码

好的,以下是使用 Python 实现的鲸鱼优化算法(Whale Optimization Algorithm)的代码示例:
```python
import numpy as np
# 定义鲸鱼优化算法类
class WOA:
def __init__(self, obj_func, dim, search_range, population_size=50, max_iter=100):
self.obj_func = obj_func # 目标函数
self.dim = dim # 变量维数
self.search_range = search_range # 变量搜索范围
self.population_size = population_size # 种群大小
self.max_iter = max_iter # 最大迭代次数
self.best_solution = None # 最优解
self.best_fitness = float("inf") # 最优适应度
self.population = np.zeros((self.population_size, self.dim)) # 种群位置
self.fitness = np.zeros(self.population_size) # 种群适应度
# 初始化种群
def init_population(self):
for i in range(self.population_size):
self.population[i] = np.random.uniform(self.search_range[0], self.search_range[1], self.dim)
self.eval_fitness()
# 计算适应度
def eval_fitness(self):
for i in range(self.population_size):
self.fitness[i] = self.obj_func(self.population[i])
if self.fitness[i] < self.best_fitness:
self.best_solution = self.population[i]
self.best_fitness = self.fitness[i]
# 鲸鱼优化算法
def optimize(self):
self.init_population()
for t in range(self.max_iter):
a = 2 - t * (2 / self.max_iter) # 衰减因子
for i in range(self.population_size):
r = np.random.uniform() # 随机数
A = 2 * a * r - a # 修正系数A
C = 2 * r # 收缩系数C
l = np.random.uniform(-1, 1, self.dim) # 随机向量l
p = np.random.uniform() # 随机概率p
if p < 0.5:
if np.abs(A) < 1:
D = np.abs(C * self.best_solution - self.population[i])
new_solution = self.best_solution - A * D * np.exp(1)**(0.5*l) # 位置更新
else:
rand_whale = self.population[np.random.randint(0, self.population_size)]
D = np.abs(C * rand_whale - self.population[i])
new_solution = rand_whale - A * D * np.exp(1)**(0.5*l) # 位置更新
else:
distance = np.abs(self.best_solution - self.population[i])
new_solution = distance * np.exp(0.5*A*l) * np.cos(2*np.pi*A*l) + self.best_solution # 位置更新
new_solution = np.clip(new_solution, self.search_range[0], self.search_range[1]) # 位置约束
new_fitness = self.obj_func(new_solution) # 计算新位置的适应度
if new_fitness < self.fitness[i]:
self.population[i] = new_solution # 更新位置
self.fitness[i] = new_fitness # 更新适应度
if new_fitness < self.best_fitness:
self.best_solution = new_solution # 更新最优解
self.best_fitness = new_fitness # 更新最优适应度
return self.best_solution, self.best_fitness
```
在使用该鲸鱼优化算法类时,需要传入目标函数、变量维数、变量搜索范围、种群大小和最大迭代次数等参数,例如:
```python
# 定义目标函数
def obj_func(x):
return x[0]**2 + x[1]**2
# 初始化鲸鱼优化算法类
woa = WOA(obj_func, dim=2, search_range=[-5, 5], population_size=50, max_iter=100)
# 运行优化算法
best_solution, best_fitness = woa.optimize()
# 输出最优解和最优适应度
print("最优解:", best_solution)
print("最优适应度:", best_fitness)
```
上述示例代码中,目标函数为 $f(x_1, x_2) = x_1^2 + x_2^2$,变量维数为2,搜索范围为 [-5, 5],种群大小为50,最大迭代次数为100。运行优化算法后输出最优解和最优适应度。
阅读全文
相关推荐

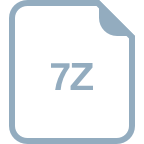
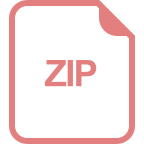
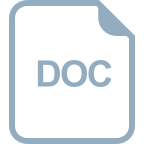
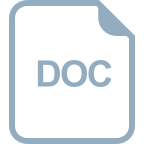







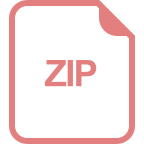
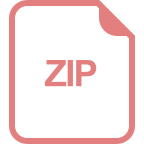