写一个机器视觉框架,包含相机连接,图像保存,通讯设置,结果显示,Log记录
时间: 2024-04-08 09:32:56 浏览: 34
下面是一个简单的机器视觉框架的示例代码,包含相机连接、图像保存、通信设置、结果显示和日志记录功能:
```python
import cv2
import logging
class MachineVisionFramework:
def __init__(self):
self.camera = None
self.result = None
self.image_save_path = "images/"
self.log_file = "log.txt"
def connect_camera(self):
try:
self.camera = cv2.VideoCapture(0) # 这里假设使用摄像头设备索引为0
logging.info("Camera connected")
except Exception as e:
logging.error(f"Failed to connect camera: {str(e)}")
def save_image(self, image):
image_path = self.image_save_path + "image.jpg"
try:
cv2.imwrite(image_path, image)
logging.info(f"Image saved to: {image_path}")
except Exception as e:
logging.error(f"Failed to save image: {str(e)}")
def set_communication(self, host, port):
try:
# 设置通信参数,例如连接远程服务器等
logging.info(f"Communication set with host: {host}, port: {port}")
except Exception as e:
logging.error(f"Failed to set communication: {str(e)}")
def display_result(self):
try:
# 显示处理结果,例如显示图像、绘制边框等
cv2.imshow("Result", self.result)
cv2.waitKey(0)
cv2.destroyAllWindows()
except Exception as e:
logging.error(f"Failed to display result: {str(e)}")
def log(self, message):
try:
logging.info(message)
except Exception as e:
print(f"Failed to log message: {str(e)}")
if __name__ == "__main__":
logging.basicConfig(filename="log.txt", level=logging.INFO)
framework = MachineVisionFramework()
framework.connect_camera()
ret, frame = framework.camera.read()
if ret:
framework.save_image(frame)
framework.set_communication("127.0.0.1", 8080)
framework.display_result()
else:
framework.log("Failed to capture image from camera")
```
在这个示例中,我们创建了一个名为MachineVisionFramework的类,它包含了连接相机、保存图像、设置通信、显示结果和记录日志的方法。在主程序中,我们首先通过调用connect_camera方法来连接相机,然后从相机中读取图像并保存。接下来,我们设置通信参数,并显示处理结果。最后,我们使用log方法记录相关信息。
请注意,这只是一个简单的示例框架,并没有实际的机器视觉算法或其他高级功能。您可以根据自己的需求扩展和修改代码。
希望这个示例对您有所帮助!如果您有任何更具体的问题,请随时提问。
阅读全文
相关推荐
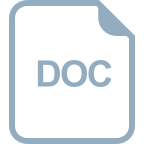
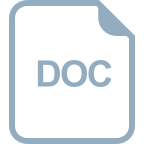
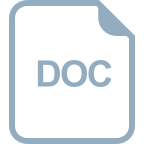
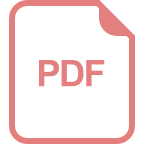
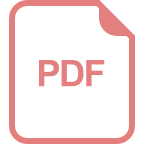
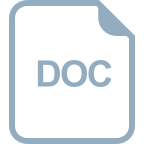
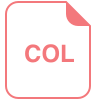
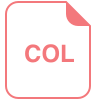
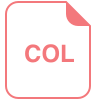
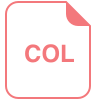
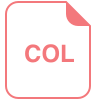
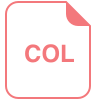
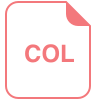
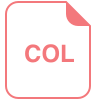
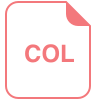
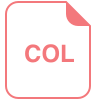
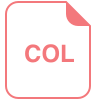
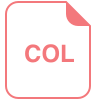