写一个spring注解AOP例子
时间: 2023-04-09 16:01:49 浏览: 156
好的,以下是一个使用Spring注解实现AOP的例子:
首先,在你的Spring配置文件中,需要开启AOP支持:
```
<bean class="org.springframework.aop.aspectj.annotation.AnnotationAwareAspectJAutoProxyCreator" />
```
然后,创建一个切面类,使用@Aspect注解标注:
```
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before executing " + joinPoint.getSignature().getName());
}
@AfterReturning(pointcut = "execution(* com.example.service.*.*(..))", returning = "result")
public void logAfterReturning(JoinPoint joinPoint, Object result) {
System.out.println("After executing " + joinPoint.getSignature().getName() + ", result is " + result);
}
@AfterThrowing(pointcut = "execution(* com.example.service.*.*(..))", throwing = "exception")
public void logAfterThrowing(JoinPoint joinPoint, Exception exception) {
System.out.println("Exception thrown from " + joinPoint.getSignature().getName() + ", exception is " + exception);
}
}
```
在上面的例子中,我们定义了三个通知,分别在方法执行前、执行后和抛出异常时执行。我们使用@Pointcut注解定义了一个切点,它匹配com.example.service包中的所有方法。然后,在通知方法上使用@Before、@AfterReturning和@AfterThrowing注解,分别表示在方法执行前、执行后和抛出异常时执行。
最后,在你的服务类中,使用@Loggable注解标注需要记录日志的方法:
```
@Service
public class MyService {
@Loggable
public void doSomething() {
// ...
}
}
```
这样,在执行doSomething方法时,LoggingAspect中定义的通知方法就会被自动调用,从而实现了AOP的功能。
阅读全文
相关推荐
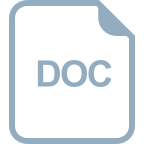
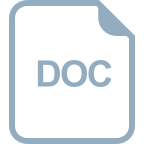
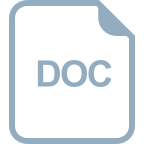

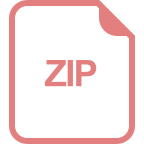
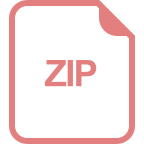
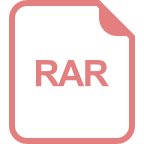
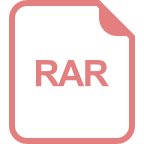
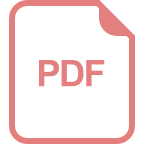
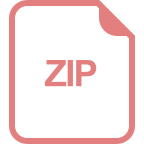
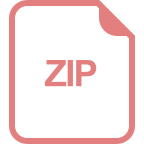
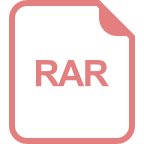
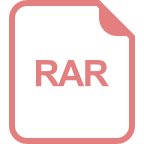
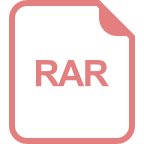
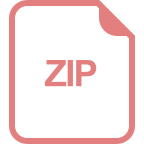
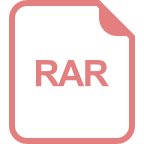
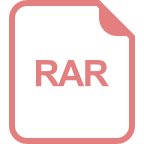
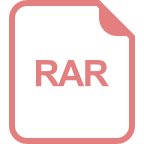
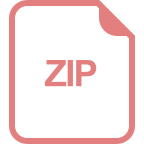