将一个二进制文件中的所有小写字母读出并复制到另一个二进制文件中去,用c++语言
时间: 2024-02-29 17:52:12 浏览: 70
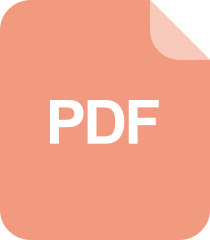
C++ 二进制读写文件的方法
可以使用 C++ 标准库中的文件流来实现这个功能。可以按照以下步骤来实现:
1. 打开原始二进制文件和目标二进制文件。
```
#include <fstream>
std::ifstream source("source.bin", std::ios::binary);
std::ofstream target("target.bin", std::ios::binary);
```
2. 循环读取原始文件中的每个字节。
```
char b;
while (source.read(&b, 1)) {
```
3. 判断字节是否为小写字母,如果是则将其写入目标文件。
```
if (std::islower(b)) {
target.write(&b, 1);
}
}
```
4. 关闭文件。
```
source.close();
target.close();
```
完整的代码如下:
```
#include <fstream>
#include <cctype>
int main() {
std::ifstream source("source.bin", std::ios::binary);
std::ofstream target("target.bin", std::ios::binary);
char b;
while (source.read(&b, 1)) {
if (std::islower(b)) {
target.write(&b, 1);
}
}
source.close();
target.close();
return 0;
}
```
请注意,在这个示例代码中,我们使用了 C++ 标准库中的文件流和字符判断函数。在实际应用中,你可能需要根据具体的需求来修改代码。
阅读全文
相关推荐
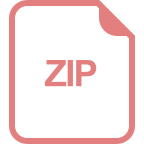
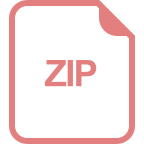
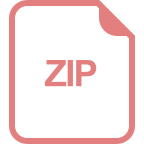
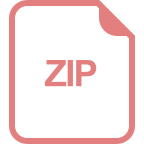
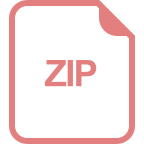
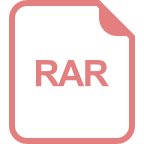
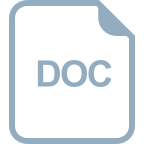
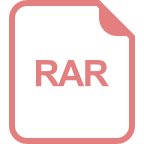
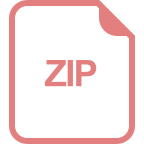
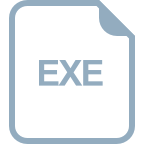
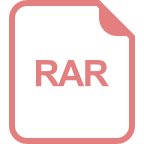
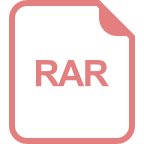
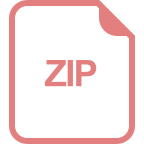
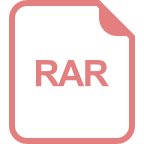