客户消费积分管理系统c语言顺序表源代码
时间: 2023-08-03 13:06:17 浏览: 288
好的,以下是一个简单的客户消费积分管理系统的 C 语言顺序表源代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 20
#define MAX_CUSTOMERS 100
typedef struct {
char name[MAX_LENGTH];
int points;
} Customer;
typedef struct {
Customer customers[MAX_CUSTOMERS];
int length;
} CustomerList;
void init_list(CustomerList *list) {
list->length = 0;
}
void add_customer(CustomerList *list, char *name, int points) {
if (list->length < MAX_CUSTOMERS) {
strcpy(list->customers[list->length].name, name);
list->customers[list->length].points = points;
list->length++;
printf("Customer added successfully\n");
} else {
printf("Customer list is full\n");
}
}
int find_customer(CustomerList *list, char *name) {
for (int i = 0; i < list->length; i++) {
if (strcmp(list->customers[i].name, name) == 0) {
return i;
}
}
return -1;
}
void add_points(CustomerList *list, char *name, int points) {
int index = find_customer(list, name);
if (index != -1) {
list->customers[index].points += points;
printf("%d points added to customer %s\n", points, name);
} else {
printf("Customer %s not found\n", name);
}
}
void subtract_points(CustomerList *list, char *name, int points) {
int index = find_customer(list, name);
if (index != -1) {
if (list->customers[index].points >= points) {
list->customers[index].points -= points;
printf("%d points subtracted from customer %s\n", points, name);
} else {
printf("Customer %s does not have enough points\n", name);
}
} else {
printf("Customer %s not found\n", name);
}
}
void print_list(CustomerList *list) {
printf("Customer List:\n");
for (int i = 0; i < list->length; i++) {
printf("%s - %d points\n", list->customers[i].name, list->customers[i].points);
}
}
int main() {
CustomerList list;
init_list(&list);
add_customer(&list, "Alice", 100);
add_customer(&list, "Bob", 200);
add_customer(&list, "Charlie", 300);
add_points(&list, "Alice", 50);
subtract_points(&list, "Bob", 100);
add_points(&list, "Dave", 50);
print_list(&list);
return 0;
}
```
该程序定义了 `Customer` 结构体和 `CustomerList` 结构体,分别表示单个客户和客户列表。程序中定义了 `init_list`、`add_customer`、`find_customer`、`add_points`、`subtract_points` 和 `print_list` 函数,用于初始化列表、添加客户、查找客户、添加积分、减少积分和打印列表。
在 `main` 函数中,我们先初始化了客户列表,然后添加了几个客户,接着对其中一些客户添加或减少了积分,最后打印了整个客户列表。
该程序只是一个简单示例,您可以在此基础上进行扩展,实现更多功能。
阅读全文
相关推荐
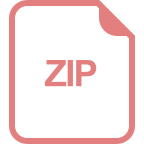
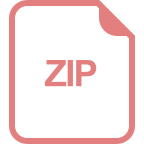















