yolo_obb中预测角度的代码
时间: 2023-05-24 21:03:52 浏览: 242
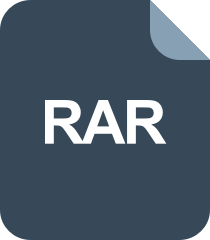
yolov7+预测代码+C++
A:yolo_obb是基于YOLOv3算法的一种目标检测模型,在进行目标检测的时候,需要预测目标框的位置、大小和角度。下面是yolo_obb中预测角度的代码实现。
首先,在模型的输出层,需要将特征图进行分离,分别预测目标框的中心点坐标、宽度和高度以及倾斜角度。代码如下:
```
def decode(conv_output, anchors, stride, num_classes, scale_xy):
num_anchors = len(anchors)
conv_shape = conv_output.shape
batch_size = conv_shape[0]
output_size = conv_shape[1:3]
conv_output = K.reshape(conv_output, (batch_size, output_size[0], output_size[1], num_anchors, 1+4+num_classes))
# 解析网络输出,得到预测的中心点、宽度、高度和倾斜角度
box_xy = K.sigmoid(conv_output[..., :2]) * scale_xy - 0.5 * (scale_xy - 1) + np.tile(range(output_size[1]), [output_size[0], 1]) * stride
box_wh = K.exp(conv_output[..., 2:4]) * anchors
box_ang = (K.sigmoid(conv_output[..., 4:5]) - 0.5) * (np.pi / 2)
# 将中心点、宽度、高度和倾斜角度转换为目标框的四个顶点
box_x1y1 = box_xy - 0.5 * box_wh
box_x2y2 = box_xy + 0.5 * box_wh
box_points = tf.concat([box_x1y1, box_x2y2, box_ang], axis=-1)
# 将目标框坐标调整为原图的比例
box_points = K.reshape(box_points, (batch_size, -1, 5))
box_points = box_points * np.array([input_shape[1], input_shape[0], input_shape[1], input_shape[0], 1])
return box_points
```
可以看到,`box_ang`即为倾斜角度的预测结果。在这里,倾斜角度的预测结果是利用Sigmoid函数将网络输出的范围调整到[-0.5,0.5]之间,然后再乘以π/2获得倾斜角度的弧度表示。可以根据需要将其转换为角度表示。
接下来,在绘制目标框时,需要将预测的倾斜角度应用于框的坐标变换。代码如下:
```
def draw_boxes(image, boxes, classes, scores):
for i, box in enumerate(boxes):
x1, y1, x2, y2, ang = box
w, h = x2 - x1, y2 - y1
# 将倾斜角度应用于目标框的坐标变换
cx, cy = x1 + w / 2, y1 + h / 2
x1 = cx + (x1 - cx) * np.cos(ang) - (y1 - cy) * np.sin(ang)
y1 = cy + (x1 - cx) * np.sin(ang) + (y1 - cy) * np.cos(ang)
x2 = cx + (x2 - cx) * np.cos(ang) - (y2 - cy) * np.sin(ang)
y2 = cy + (x2 - cx) * np.sin(ang) + (y2 - cy) * np.cos(ang)
# 绘制目标框
cv2.rectangle(image, (int(x1), int(y1)), (int(x2), int(y2)), (255, 0, 0), 2)
cv2.putText(image, '{} {:.2f}'.format(classes[i], scores[i]),
(int(x1), int(y1 - 5)), cv2.FONT_HERSHEY_SIMPLEX, 0.5,
(255, 0, 0), 2, cv2.LINE_AA)
```
可以看到,在变换坐标时,倾斜角度的余弦和正弦值被用于旋转变换。最后,再将变换后的坐标用于绘制目标框。
阅读全文
相关推荐
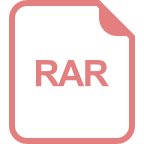
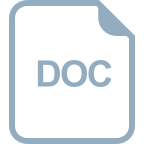
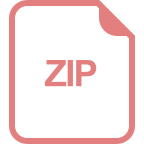
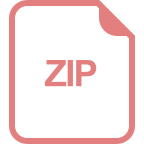
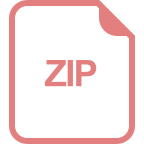
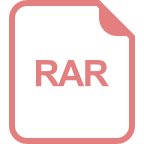
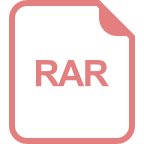
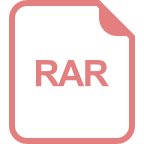
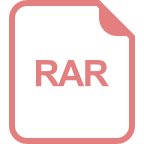
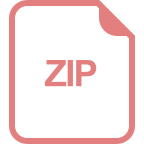
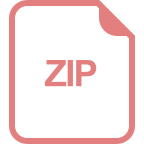
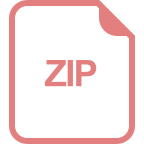
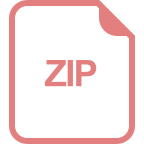
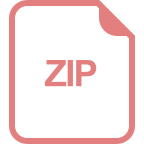
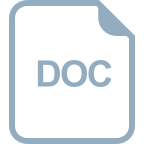
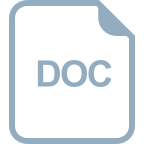