java opencv 调用摄像头_opencv java实现调用摄像头动态识别人脸
时间: 2023-07-04 17:26:12 浏览: 106
好的,以下是使用 Java OpenCV 库实现调用摄像头动态识别人脸的代码示例:
```java
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.core.MatOfRect;
import org.opencv.core.Point;
import org.opencv.core.Rect;
import org.opencv.core.Scalar;
import org.opencv.core.Size;
import org.opencv.imgproc.Imgproc;
import org.opencv.objdetect.CascadeClassifier;
import org.opencv.videoio.VideoCapture;
public class FaceRecognition {
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
CascadeClassifier faceDetector = new CascadeClassifier("haarcascade_frontalface_alt.xml");
VideoCapture capture = new VideoCapture(0);
MatOfRect faceDetections = new MatOfRect();
Mat frame = new Mat();
while (capture.read(frame)) {
Imgproc.cvtColor(frame, frame, Imgproc.COLOR_BGR2GRAY);
Imgproc.equalizeHist(frame, frame);
faceDetector.detectMultiScale(frame, faceDetections, 1.1, 3, 0, new Size(30, 30), new Size());
for (Rect rect : faceDetections.toArray()) {
Imgproc.rectangle(frame, new Point(rect.x, rect.y), new Point(rect.x + rect.width, rect.y + rect.height), new Scalar(0, 255, 0), 2);
}
HighGui.imshow("Face Detection", frame);
if (HighGui.waitKey(1) == 27) {
break;
}
}
capture.release();
HighGui.destroyAllWindows();
}
}
```
代码中使用了 OpenCV 自带的人脸检测分类器 `haarcascade_frontalface_alt.xml` 进行人脸检测。通过 `VideoCapture(0)` 调用摄像头进行图像采集,并使用 `while` 循环处理采集到的每一帧图像。
在每一帧图像中,首先将图像转换为灰度图像,并使用直方图均衡化处理,以增强人脸检测的效果。然后使用 `faceDetector.detectMultiScale()` 方法进行人脸检测,返回一个 `MatOfRect` 类型的结果,其中包含检测到的所有人脸的矩形框。
最后,遍历每个矩形框,并使用 `Imgproc.rectangle()` 方法在原图像中画出矩形框,以显示检测结果。同时使用 `HighGui.imshow()` 方法将处理后的图像显示在窗口中,并使用 `HighGui.waitKey()` 方法等待用户按下 ESC 键结束程序。
希望这段代码能对你有所帮助。
阅读全文
相关推荐
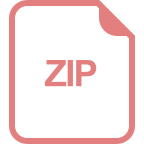
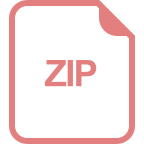
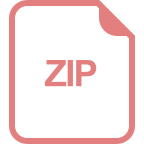
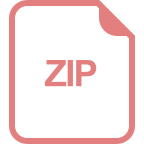
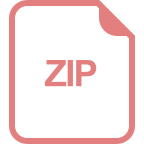
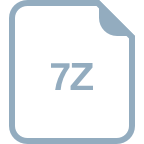
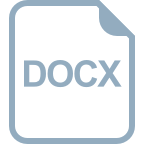
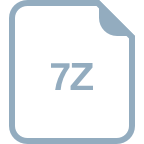