for i, params in enumerate(grid_search.param_grid): print(f"Testing combination {i+1}/{len(grid_search.param_grid)}: {params}") grid_search.fit(X_train, y_train) print(f"Score: {grid_search.best_score_:.3f}\n")
时间: 2024-02-16 11:03:19 浏览: 41
这段代码的作用是进行网格搜索,并在每个参数组合完成验证之后输出评分。具体来说,这段代码的执行过程如下:
1. 使用 `enumerate()` 函数遍历 `grid_search.param_grid` 中的每一个参数组合。`i` 表示当前参数组合的索引,`params` 表示当前参数组合的取值。
2. 输出当前正在测试的参数组合的信息,包括当前参数组合的索引、总共需要测试的参数组合数量,以及当前参数组合的取值。
```
print(f"Testing combination {i+1}/{len(grid_search.param_grid)}: {params}")
```
3. 使用当前参数组合对 `grid_search` 进行拟合,并在训练集和验证集上进行交叉验证。
```
grid_search.fit(X_train, y_train)
```
4. 输出当前参数组合在训练集上的交叉验证分数。
```
print(f"Score: {grid_search.best_score_:.3f}\n")
```
其中,`grid_search.best_score_` 表示当前最优的交叉验证分数。在每个参数组合完成验证之后,我们都会输出当前参数组合的交叉验证分数,以便我们及时了解当前参数组合的效果。
相关问题
解释for i ,content in enumerate(path_list): img, xml = content text = img + ' ' + xml + '\n' if i < len(path_list) * ratio: train_f.write(text) else: val_f.write(text) train_f.close() val_f.close()
这段代码是将一个包含图片和xml文件路径的列表`path_list`分成训练集和验证集,并将路径写入对应的文件中。具体解释如下:
1. `enumerate(path_list)`用于遍历列表`path_list`中的元素,返回元素在列表中的索引和元素本身,分别赋值给`i`和`content`。
2. `img, xml = content`将元素`content`中的图片路径和xml文件路径分别赋值给变量`img`和`xml`。
3. `text = img + ' ' + xml + '\n'`将图片路径和xml文件路径拼接成一个字符串,并在末尾添加一个换行符,保存在变量`text`中。
4. `if i < len(path_list) * ratio:`如果当前元素的索引`i`小于`path_list`长度乘以比例`ratio`,则将`text`写入训练集文件中;否则将`text`写入验证集文件中。这里假设比例`ratio`是0.8,即训练集占总数据集的80%,验证集占20%。
5. `train_f.close()`和`val_f.close()`关闭文件句柄,确保写入操作已经完成并且文件已经被保存。
综上所述,这段代码的作用是将一个包含图片和xml文件路径的列表分成训练集和验证集,并将路径写入对应的文件中。其中,训练集和验证集的比例可以通过`ratio`参数自定义。
for i, (k, v) in enumerate(centroids_state_dict.items()):
这是一个Python代码片段,使用了内置函数enumerate和items()方法。具体解释如下:
- centroids_state_dict是一个字典类型的变量,包含键值对。
- items()方法返回字典中所有键值对的元组。
- for循环中的(i, (k, v)),i是enumerate函数返回的索引,(k, v)是字典中的键值对元组。
- 循环中的代码可以提取出字典中每一个键值对的键k和值v,并且对它们进行操作。
相关推荐
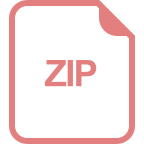













