如何用springboot实现翻译小程序服务端
时间: 2023-11-28 19:51:39 浏览: 78
要使用Spring Boot实现翻译小程序服务端,可以按照以下步骤:
1. 首先,需要选择一种翻译API,比如百度翻译API、Google翻译API等。在这里,我们以百度翻译API为例。
2. 在项目中引入相关的依赖,比如Spring Boot、Spring Web、Spring Boot Starter Test等。可以在pom.xml文件中添加以下依赖:
```
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.baidu</groupId>
<artifactId>baidu-translate-api</artifactId>
<version>3.15.0</version>
</dependency>
</dependencies>
```
3. 在application.properties中配置百度翻译API的相关信息,包括app id、密钥等。
```
baidu.translate.appid=your_app_id
baidu.translate.securityKey=your_security_key
```
4. 创建一个Controller,来处理翻译请求。可以使用@RestController注解来标记这个Controller。
```
@RestController
public class TranslateController {
@Autowired
private BaiduTranslateService baiduTranslateService;
@PostMapping("/translate")
public String translate(@RequestParam String q, @RequestParam String from, @RequestParam String to) {
return baiduTranslateService.translate(q, from, to);
}
}
```
5. 创建一个Service,来调用百度翻译API。可以使用@Autowired注解来注入BaiduTranslate类。
```
@Service
public class BaiduTranslateService {
@Autowired
private BaiduTranslate baiduTranslate;
public String translate(String q, String from, String to) {
return baiduTranslate.translate(q, from, to);
}
}
```
6. 编写一个测试类,测试翻译功能是否正常。
```
@SpringBootTest
class TranslateApplicationTests {
@Autowired
private BaiduTranslateService baiduTranslateService;
@Test
void testTranslate() {
String q = "Hello World";
String from = "en";
String to = "zh";
String result = baiduTranslateService.translate(q, from, to);
System.out.println(result);
}
}
```
以上就是使用Spring Boot实现翻译小程序服务端的基本步骤。当然,还需要根据具体需求进行扩展和优化。
阅读全文
相关推荐
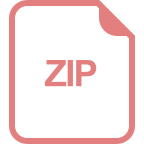
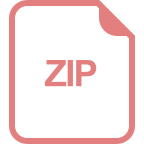

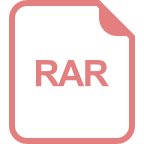
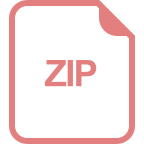
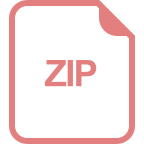
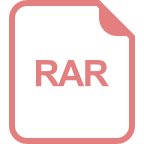
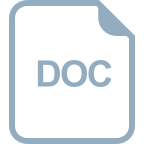
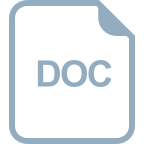

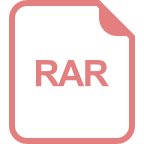
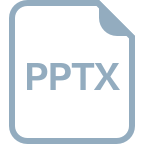
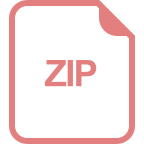
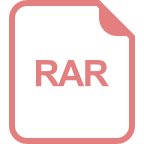
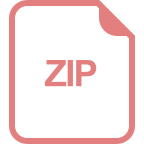
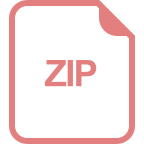
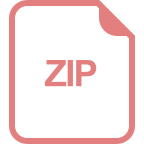
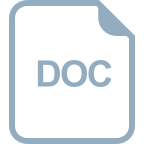