java解析音乐频谱
时间: 2023-06-29 09:13:02 浏览: 96
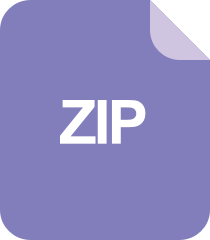
java对音频文件的频谱分析

要解析音乐频谱,可以使用Java中的音频处理库,如Java Sound API或JFugue。以下是一些步骤:
1. 从音频文件中读取音频数据。
2. 应用FFT(快速傅里叶变换)算法将音频数据转换为频域数据。
3. 将频域数据绘制成频谱图。
下面是一个简单的示例代码,使用Java Sound API解析音乐频谱:
```java
import javax.sound.sampled.*;
import java.io.*;
public class AudioSpectrumAnalyzer {
public static void main(String[] args) {
try {
// 读取音频文件
AudioInputStream audioInputStream = AudioSystem.getAudioInputStream(new File("music.wav"));
// 获取音频格式
AudioFormat audioFormat = audioInputStream.getFormat();
// 创建数据行
DataLine.Info info = new DataLine.Info(SourceDataLine.class, audioFormat);
SourceDataLine dataLine = (SourceDataLine) AudioSystem.getLine(info);
// 打开数据行
dataLine.open(audioFormat);
dataLine.start();
// 读取音频数据
int bufferSize = (int) audioFormat.getSampleRate() * audioFormat.getFrameSize();
byte[] buffer = new byte[bufferSize];
int bytesRead = 0;
while (bytesRead != -1) {
bytesRead = audioInputStream.read(buffer, 0, buffer.length);
if (bytesRead >= 0) {
// 将音频数据写入数据行
dataLine.write(buffer, 0, bytesRead);
// 解析频谱数据
float[] spectrum = getFrequencySpectrum(buffer, audioFormat);
// 在控制台中打印频谱数据
for (int i = 0; i < spectrum.length; i++) {
System.out.print(spectrum[i] + " ");
}
System.out.println();
}
}
// 关闭数据行和音频输入流
dataLine.drain();
dataLine.stop();
dataLine.close();
audioInputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
// 获取频谱数据
private static float[] getFrequencySpectrum(byte[] audioData, AudioFormat audioFormat) {
// 将字节数组转换为16位整数数组
int[] audioSamples = new int[audioData.length / 2];
for (int i = 0, j = 0; i < audioData.length; i += 2, j++) {
audioSamples[j] = ((audioData[i + 1] & 0xff) << 8) | (audioData[i] & 0xff);
}
// 创建FFT对象
FFT fft = new FFT(audioSamples.length);
// 应用FFT变换
fft.forward(audioSamples);
// 获取频域数据
float[] spectrum = new float[audioSamples.length / 2 + 1];
for (int i = 0; i < spectrum.length; i++) {
// 计算频率
float frequency = i * audioFormat.getSampleRate() / audioSamples.length;
// 计算幅度
float amplitude = (float) Math.sqrt(fft.real[i] * fft.real[i] + fft.imag[i] * fft.imag[i]);
// 将幅度归一化到0到1之间
amplitude = amplitude / audioSamples.length;
spectrum[i] = amplitude;
}
return spectrum;
}
// FFT类
private static class FFT {
int n, m;
float[] real, imag;
public FFT(int n) {
this.n = n;
this.m = (int) (Math.log(n) / Math.log(2));
this.real = new float[n];
this.imag = new float[n];
}
public void forward(int[] x) {
for (int i = 0; i < n; i++) {
real[i] = (float) x[i];
imag[i] = 0;
}
int h = 0;
for (int i = 0; i < n - 1; i++) {
if (i < h) {
float tr = real[h];
float ti = imag[h];
real[h] = real[i];
imag[h] = imag[i];
real[i] = tr;
imag[i] = ti;
}
int k = n / 2;
while (k <= h) {
h -= k;
k /= 2;
}
h += k;
}
for (int r = 1; r <= m; r++) {
int p = (int) Math.pow(2, r);
float s = 1;
float t = (float) Math.cos(Math.PI / p);
float u = (float) Math.sin(Math.PI / p);
for (int k = 0; k < n; k += p) {
float tempReal = 1;
float tempImag = 0;
for (int l = k; l < k + p / 2; l++) {
float vr = real[l + p / 2] * tempReal - imag[l + p / 2] * tempImag;
float vi = real[l + p / 2] * tempImag + imag[l + p / 2] * tempReal;
real[l + p / 2] = real[l] - vr;
imag[l + p / 2] = imag[l] - vi;
real[l] += vr;
imag[l] += vi;
float temp = tempReal;
tempReal = tempReal * t - tempImag * u;
tempImag = temp * u + tempImag * t;
}
s *= t;
}
}
}
}
}
```
这段代码读取名为“music.wav”的音频文件,解析其频谱数据,并在控制台中打印输出。可以根据自己的需要修改代码以实现更复杂的音频处理任务。
阅读全文
相关推荐

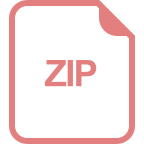
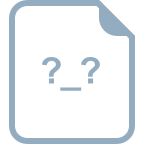
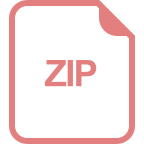
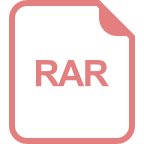
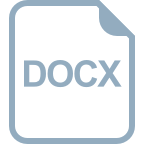
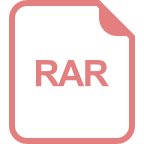
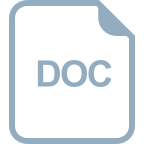
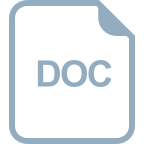
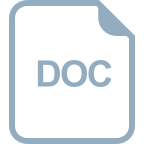
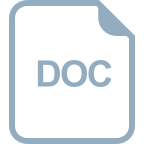
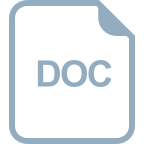
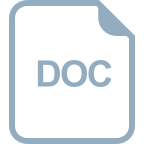
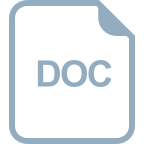
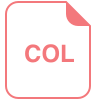
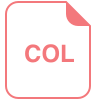