ts类的修饰符
时间: 2023-07-30 11:09:09 浏览: 102
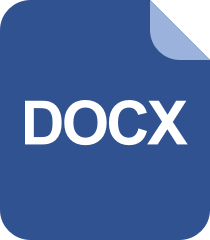
类修饰符大全
TypeScript中类的修饰符有以下几种:
1. public
public是默认修饰符,即不写修饰符时默认为public。public修饰的属性或方法可以在类的内部和外部使用。
2. private
private修饰的属性或方法只能在类的内部使用,无法在类的外部使用。
3. protected
protected修饰的属性或方法可以在类的内部和子类中使用,但无法在类的外部使用。
4. readonly
readonly修饰的属性只能在初始化时或构造函数中赋值,之后就无法再修改。
示例代码:
```typescript
class Person {
public name: string; // 公共属性
private age: number; // 私有属性
protected gender: string; // 保护属性
readonly id: number; // 只读属性
constructor(name: string, age: number, gender: string, id: number) {
this.name = name;
this.age = age;
this.gender = gender;
this.id = id;
}
public sayHello() { // 公共方法
console.log(`Hello, my name is ${this.name}.`);
}
private getAge() { // 私有方法
return this.age;
}
protected getGender() { // 保护方法
return this.gender;
}
}
class Student extends Person {
public getGenderAndAge() {
console.log(`My gender is ${this.getGender()} and age is ${this.getAge()}.`);
}
}
const person = new Person('Tom', 18, 'male', 1001);
console.log(person.name); // Tom
// console.log(person.age); // 无法访问
// console.log(person.gender); // 无法访问
// person.id = 1002; // 无法修改
person.sayHello(); // Hello, my name is Tom.
const student = new Student('Jerry', 17, 'female', 1002);
// console.log(student.age); // 无法访问
// console.log(student.gender); // 无法访问
console.log(student.name); // Jerry
console.log(student.id); // 1002
student.getGenderAndAge(); // My gender is female and age is undefined.
```
在上面的示例代码中,我们定义了一个Person类,其中包含了公共属性、私有属性、保护属性、只读属性、公共方法、私有方法和保护方法。这些属性和方法都有不同的修饰符,可以在不同的场景下使用。同时,我们还定义了一个Student类,继承自Person类,可以访问Person类中的保护属性和保护方法。
阅读全文
相关推荐
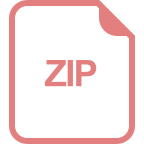
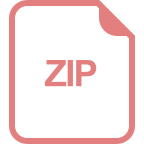

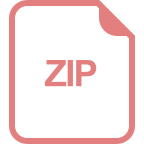
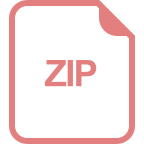
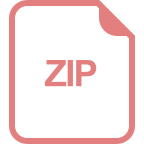
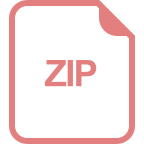
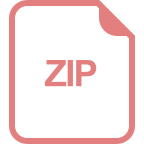
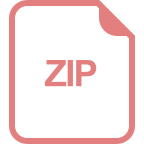
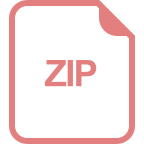
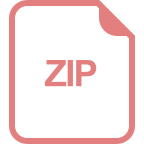
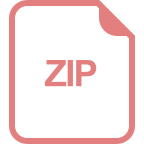
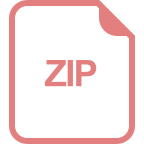
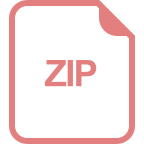
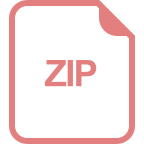
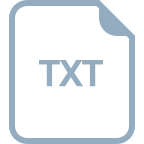
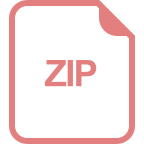
