以上所解答的,使用Java语言实现
时间: 2024-02-23 14:59:03 浏览: 81
好的,使用Java语言实现校验邮箱客户端账号密码是否正确,可以使用JavaMail API提供的IMAP、POP3或SMTP协议进行验证。具体实现如下:
```
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.util.Properties;
public class EmailAuthentication {
public static boolean validateCredentials(String email, String password, String host, String protocol) {
Properties properties = new Properties();
properties.put("mail." + protocol + ".host", host);
properties.put("mail." + protocol + ".port", "587");
properties.put("mail." + protocol + ".auth", "true");
properties.put("mail." + protocol + ".starttls.enable", "true");
Session session = Session.getInstance(properties, new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(email, password);
}
});
try {
Transport transport = session.getTransport(protocol);
transport.connect();
transport.close();
System.out.println("Authentication successful.");
return true;
} catch (MessagingException e) {
System.out.println("Authentication failed: " + e.getMessage());
return false;
}
}
public static void main(String[] args) {
String email = "your_email@example.com";
String password = "your_password";
String host = "mail.example.com";
String protocol = "imap"; // or "pop3" or "smtp"
validateCredentials(email, password, host, protocol);
}
}
```
在主函数中,分别定义了要验证的邮箱账号、密码、邮箱服务器地址和协议。调用`validateCredentials()`方法传入这些参数即可进行验证。该方法内部使用JavaMail API提供的`Session`和`Transport`类进行连接和验证。如果验证成功,将会打印`Authentication successful.`,否则将会打印`Authentication failed: `和错误信息。
阅读全文
相关推荐
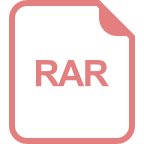
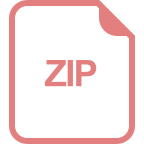
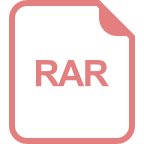
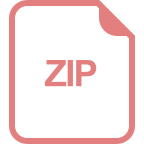
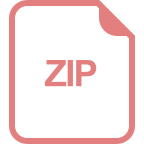
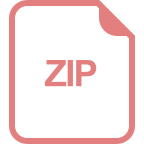
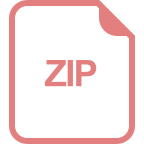
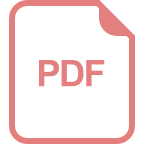
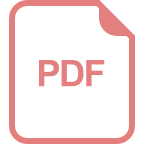
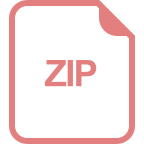
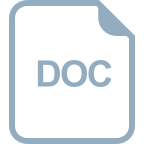
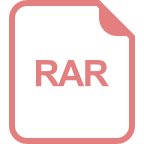
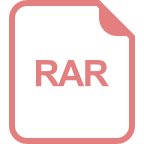