使用STL分别按照学生的身高和成绩进行排序
时间: 2023-08-08 17:14:05 浏览: 128
按照学生的身高进行排序可以使用STL中的sort函数,示例代码如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Student {
string name;
int height;
int score;
};
bool cmp_height(const Student& a, const Student& b) {
return a.height > b.height;
}
int main() {
vector<Student> students = {
{"Tom", 180, 90},
{"Jerry", 170, 80},
{"Alice", 175, 95},
{"Bob", 190, 85}
};
sort(students.begin(), students.end(), cmp_height);
for (auto& student : students) {
cout << student.name << " " << student.height << " " << student.score << endl;
}
return 0;
}
```
按照学生的成绩进行排序同样可以使用sort函数,只需要将比较函数改为按照成绩进行比较即可,示例代码如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Student {
string name;
int height;
int score;
};
bool cmp_score(const Student& a, const Student& b) {
return a.score > b.score;
}
int main() {
vector<Student> students = {
{"Tom", 180, 90},
{"Jerry", 170, 80},
{"Alice", 175, 95},
{"Bob", 190, 85}
};
sort(students.begin(), students.end(), cmp_score);
for (auto& student : students) {
cout << student.name << " " << student.height << " " << student.score << endl;
}
return 0;
}
```
阅读全文
相关推荐
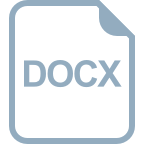
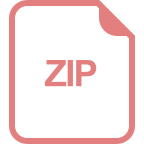
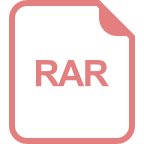
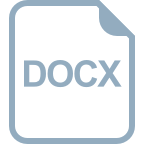
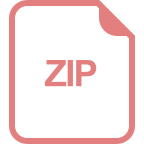
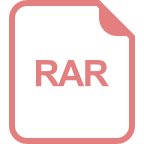
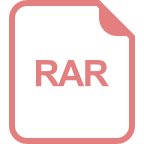
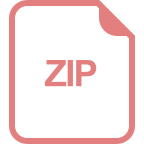
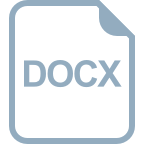
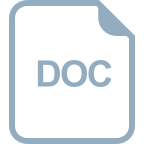
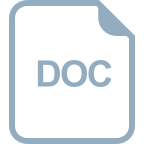
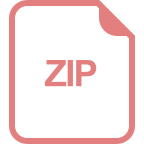
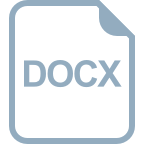
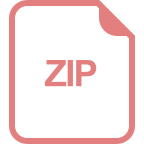
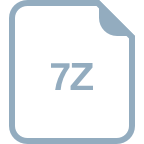