如何在PyQt5中创建并设置一个状态栏来显示当前日期?
时间: 2024-11-24 16:40:47 浏览: 5
在PyQt5中,你可以按照以下步骤来创建并设置一个状态栏来显示当前日期:
1. 导入所需的库:
```python
from PyQt5.QtWidgets import QMainWindow, QApplication, QStatusBar, QLabel
from PyQt5.QtCore import Qt, QDateTime
```
2. 创建主窗口(QMainWindow):
```python
class MainWindow(QMainWindow):
def __init__(self):
super(MainWindow, self).__init__()
```
3. 设置UI布局,包括创建状态栏(QStatusBar):
```python
def setupUi(self):
self.setWindowTitle('PyQt5 Status Bar Example')
self.setGeometry(100, 100, 800, 600)
self.statusBar() # 创建状态栏,无需显式实例化
```
4. 定义一个槽函数(slot),用于定时更新状态栏:
```python
@Slot()
def updateStatusBar(self):
current_date = QDateTime.currentDateTime().toString(Qt.ISODate) # 获取当前日期,格式化为ISO标准
self.statusBar().showMessage(current_date) # 更新状态栏内容
```
5. 在主函数里启动应用,并在适当的地方设置更新间隔:
```python
if __name__ == '__main__':
app = QApplication([])
main_window = MainWindow()
main_window.updateStatusBar() # 首次显示当前日期
timer = QTimer(main_window) # 创建一个计时器
timer.timeout.connect(main_window.updateStatusBar) # 每隔一段时间调用updateStatusBar
timer.start(1000) # 每秒更新一次
main_window.show()
app.exec_()
```
这样,每当应用程序运行时,状态栏就会每秒更新显示当前日期。如果你想改变更新频率,只需调整`start`函数中的参数即可。
阅读全文
相关推荐
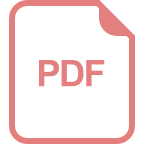
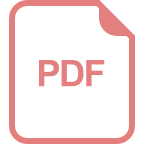
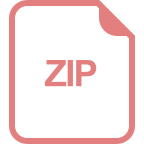















