c语言小游戏代码飞机大战
时间: 2024-10-09 09:02:39 浏览: 18
飞机大战是一款经典的小游戏,它的代码实现相对简单,非常适合初学者练手。
游戏的主要思路是玩家控制一个飞机,通过发射子弹和躲避敌机的攻击来生存,游戏难度逐渐升级。以下是一个简单的C语言实现飞机大战的代码框架:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <windows.h>
#define WIDTH 50
#define HEIGHT 30
int score = 0; //分数
void gotoxy(int x, int y); //光标跳转函数
void draw_plane(int x, int y); //画出飞机
void draw_bullet(int x, int y); //画出子弹
void draw_enemy(int x, int y); //画出敌机
void update_bullet(int bullet_x[], int bullet_y[]); //更新子弹位置
void update_enemy(int enemy_x[], int enemy_y[]); //更新敌机位置
void check_collision(int bullet_x[], int bullet_y[], int enemy_x[], int enemy_y[]); //检查碰撞情况
int main()
{
char ch = ' ';
int i, j;
int plane_x = WIDTH / 2, plane_y = HEIGHT - 2; //初始飞机位置
int bullet_x[10] = {0}, bullet_y[10] = {0}; //子弹的位置数组
int enemy_x[10] = {0}, enemy_y[10] = {0}; //敌机的位置数组
while (ch != 'q')
{
system("cls"); //清屏
gotoxy(0, 0);
printf("Score:%d\n", score); //打印分数
for (i = 0; i < WIDTH; i++)
printf("#"); //画边框
printf("\n");
for (i = 0; i < HEIGHT; i++)
{
for (j = 0; j < WIDTH; j++)
{
if ((j == 0) || (j == WIDTH - 1))
printf("#"); //画边框
else if ((i == plane_y) && (j == plane_x))
draw_plane(plane_x, plane_y); //画飞机
else if ((j == bullet_x[i]) && (i == bullet_y[i]))
draw_bullet(bullet_x[i], bullet_y[i]); //画子弹
else if ((j == enemy_x[i]) && (i == enemy_y[i]))
draw_enemy(enemy_x[i], enemy_y[i]); //画敌机
else
printf(" ");
}
printf("\n");
}
for (i = 0; i < 10; i++)
{
if (bullet_y[i] > 0)
bullet_y[i]--;
if (enemy_y[i] > 0)
enemy_y[i]++;
else
{
enemy_y[i] = 1;
enemy_x[i] = rand() % (WIDTH - 2) + 1;
}
}
if (kbhit()) //判断是否有键盘输入
{
ch = getch();
if (ch == 'a' && plane_x > 1)
plane_x--;
if (ch == 'd' && plane_x < WIDTH - 2)
plane_x++;
if (ch == 'w' && plane_y > 1)
plane_y--;
if (ch == 's' && plane_y < HEIGHT - 2)
plane_y++;
if (ch == ' ')
{
for (i = 0; i < 10; i++)
{
if (bullet_y[i] == 0)
{
bullet_x[i] = plane_x;
bullet_y[i] = plane_y - 1;
break;
}
}
}
}
update_bullet(bullet_x, bullet_y); //更新子弹位置
update_enemy(enemy_x, enemy_y); //更新敌机位置
check_collision(bullet_x, bullet_y, enemy_x, enemy_y); //检查碰撞情况
Sleep(50); //暂停50毫秒,控制游戏速度
}
return 0;
}
void gotoxy(int x, int y)
{
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw_plane(int x, int y)
{
gotoxy(x, y);
printf("^");
gotoxy(x - 1, y + 1);
printf("<-0->");
}
void draw_bullet(int x, int y)
{
gotoxy(x, y);
printf("|");
}
void draw_enemy(int x, int y)
{
gotoxy(x, y);
printf("X");
}
void update_bullet(int bullet_x[], int bullet_y[])
{
int i;
for (i = 0; i < 10; i++)
{
if (bullet_y[i] > 0)
{
gotoxy(bullet_x[i], bullet_y[i] + 1);
printf(" ");
gotoxy(bullet_x[i], bullet_y[i]);
printf("|");
}
}
}
void update_enemy(int enemy_x[], int enemy_y[])
{
int i;
for (i = 0; i < 10; i++)
{
if (enemy_y[i] > 0)
{
gotoxy(enemy_x[i], enemy_y[i] - 1);
printf(" ");
gotoxy(enemy_x[i], enemy_y[i]);
printf("X");
}
}
}
void check_collision(int bullet_x[], int bullet_y[], int enemy_x[], int enemy_y[])
{
int i, j;
for (i = 0; i < 10; i++)
{
if (bullet_y[i] > 0)
{
for (j = 0; j < 10; j++)
{
if ((bullet_x[i] == enemy_x[j]) && (bullet_y[i] == enemy_y[j]))
{
score++;
gotoxy(enemy_x[j], enemy_y[j]);
printf(" ");
bullet_x[i] = 0;
bullet_y[i] = 0;
enemy_x[j] = rand() % (WIDTH - 2) + 1;
enemy_y[j] = 1;
}
}
}
}
}
```
以上是一个简单的C语言实现飞机大战的代码框架,你可以根据自己的理解进行修改和完善。
阅读全文
相关推荐
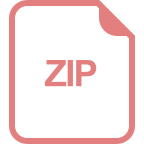
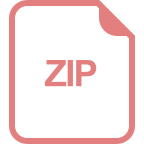
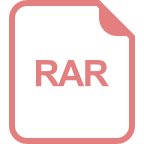
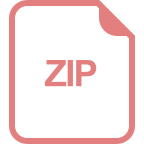
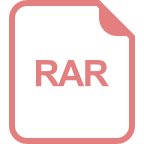
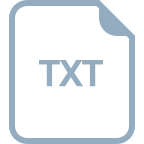
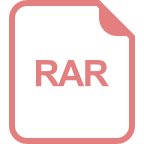
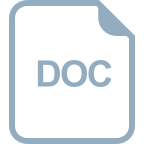
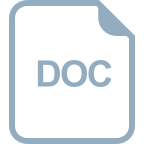
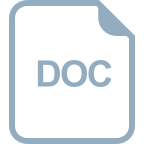




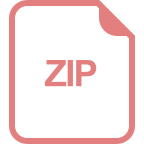