在jsp中如何进行like过滤,举例,含义
时间: 2024-10-20 13:09:10 浏览: 19
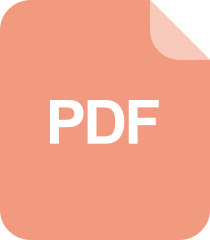
JSP利用过滤器解决request中文乱码问题
在JSP(Java Server Pages)中进行LIKE过滤通常涉及到使用JDBC(Java Database Connectivity)连接数据库,特别是当你要对用户输入进行模糊搜索时。例如,假设你有一个包含用户姓名的列表,你想让用户通过输入名字的一部分(如前几个字母或姓氏)来查找匹配的记录。
首先,你需要获取用户的输入,这通常通过表单提交来实现:
```jsp
<form action="search.jsp" method="post">
<input type="text" name="keyword" placeholder="请输入要搜索的名字...">
<input type="submit" value="搜索">
</form>
```
接着,在search.jsp中,我们可以使用PreparedStatement来防止SQL注入攻击,并执行LIKE查询:
```jsp
<%@ page import="java.sql.*" %>
<%!
Connection conn = null;
PreparedStatement pstmt = null;
%>
<%
String keyword = request.getParameter("keyword"); // 获取用户输入
try {
// 连接到数据库
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/your_database", "username", "password");
// 准备SQL语句,使用百分号通配符 '%'
String sql = "SELECT * FROM users WHERE name LIKE ?";
pstmt = conn.prepareStatement(sql);
// 设置通配符和用户输入
pstmt.setString(1, "%" + keyword + "%");
ResultSet rs = pstmt.executeQuery();
// 显示结果
while (rs.next()) {
out.println(rs.getString("name")); // 或者其他字段名
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
%>
```
这个例子中,`%" + keyword + "%"`部分表示将用户输入前后各加上一个百分号,使得`LIKE`操作能够匹配任意字符,包括用户输入的部分。如果用户输入的是"John",那么查询会返回所有名字中包含"John"的记录,不论是在开头、结尾还是中间。
阅读全文
相关推荐
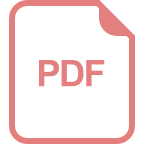
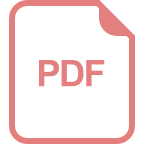
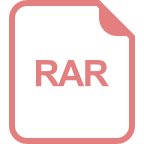
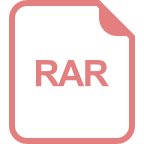
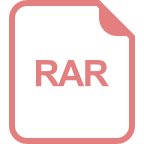
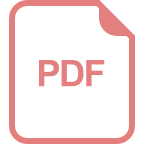
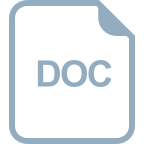
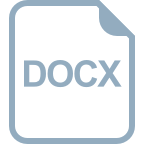
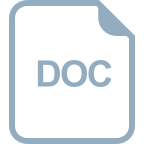
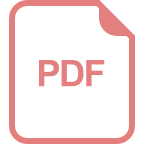
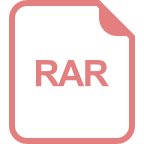
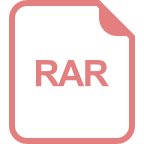
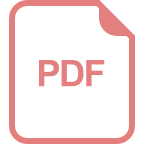
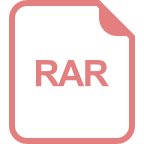
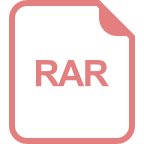
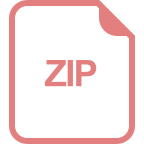
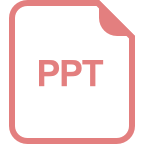
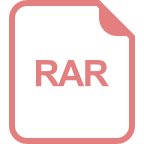