python访问远程主机时,正在连接,但还为连接上关闭程序时,把连接的线程也关闭
时间: 2024-10-20 20:10:45 浏览: 30
在Python中,当尝试通过socket或其他网络库访问远程主机时,可能会创建一个单独的线程来处理网络通信。如果你需要在中断操作(例如用户请求退出或程序意外关闭)时确保网络连接也被安全地断开,可以使用以下策略:
1. **使用异步编程**:Python的`asyncio`库允许你编写异步代码,其中包含了协程和事件循环的概念。你可以使用`asyncio.sleep()`结合`KeyboardInterrupt`异常来优雅地处理这种情况,如在连接时添加一个`try-except`块,并在接收到`KeyboardInterrupt`时关闭连接。
```python
import asyncio
async def connect_and_close():
while True:
try:
await connect_to_host()
break
except KeyboardInterrupt:
close_connection()
asyncio.run(connect_and_close())
```
2. **使用`threading`的`Thread`或`concurrent.futures`**:如果在传统的线程环境中,可以设置一个标志变量,在主线程中检测这个标志,然后在线程内部定期检查该标志并断开连接。
```python
from threading import Thread, Event
class ConnectionThread(Thread):
def __init__(self, event):
super().__init__()
self.event = event
self.conn = None
def run(self):
try:
self.conn = establish_connection()
while not self.event.is_set():
# 检查连接是否应该关闭
if self.conn and should_close_connection():
self.conn.close()
self.conn = None
except KeyboardInterrupt:
# 如果有异常,也要确保关闭连接
if self.conn:
self.conn.close()
thread = ConnectionThread(threading.Event())
thread.start()
# 主线程处理中断信号,触发event
thread.event.set()
```
无论哪种方法,都要确保在适当的时机(比如接收到中断信号)关闭资源,避免资源泄漏。记得清理已经打开的套接字、数据库连接等,以释放系统资源。
阅读全文
相关推荐
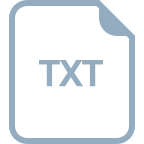
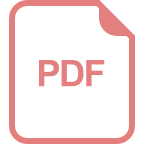
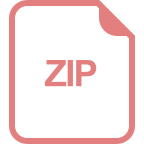















