仿写 linux 下的 cp 命令
时间: 2024-05-16 14:10:01 浏览: 114
在 Linux 下,cp 命令是用于复制文件或目录的命令,其基本语法格式如下:
```
cp [选项] 源文件或目录 目标文件或目录
```
其中,源文件或目录为需要复制的文件或目录,目标文件或目录为复制后的文件或目录。
常用的选项有:
- -r:递归复制目录及其子目录中的所有文件。
- -f:覆盖已存在的目标文件而不询问用户。
- -i:如果目标文件已存在,提示用户是否覆盖。
- -v:显示复制进度和详细信息。
以下是仿写 cp 命令的示例代码,实现了复制单个文件和递归复制目录的功能:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/stat.h>
#define BUF_SIZE 4096
void copy_file(char *src, char *dst);
void copy_dir(char *src, char *dst);
int main(int argc, char *argv[])
{
if (argc != 3) {
printf("Usage: %s <source> <destination>\n", argv);
exit(1);
}
struct stat src_stat;
if (stat(argv, &src_stat) == -1) {
perror("stat");
exit(1);
}
if (S_ISREG(src_stat.st_mode)) {
copy_file(argv, argv);
} else if (S_ISDIR(src_stat.st_mode)) {
copy_dir(argv, argv);
} else {
printf("%s is not a regular file or directory\n", argv);
exit(1);
}
printf("Copy completed!\n");
exit(0);
}
void copy_file(char *src, char *dst)
{
int fd_src, fd_dst, n;
char buf[BUF_SIZE];
if ((fd_src = open(src, O_RDONLY)) == -1) {
perror("open");
exit(1);
}
if ((fd_dst = open(dst, O_WRONLY | O_CREAT | O_TRUNC, 0644)) == -1) {
perror("open");
exit(1);
}
while ((n = read(fd_src, buf, BUF_SIZE)) > 0) {
if (write(fd_dst, buf, n) != n) {
perror("write");
exit(1);
}
}
close(fd_src);
close(fd_dst);
}
void copy_dir(char *src, char *dst)
{
DIR *dir;
struct dirent *entry;
struct stat src_stat;
char src_path[PATH_MAX], dst_path[PATH_MAX];
if ((dir = opendir(src)) == NULL) {
perror("opendir");
exit(1);
}
if (mkdir(dst, 0755) == -1 && errno != EEXIST) {
perror("mkdir");
exit(1);
}
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0)
continue;
snprintf(src_path, PATH_MAX, "%s/%s", src, entry->d_name);
snprintf(dst_path, PATH_MAX, "%s/%s", dst, entry->d_name);
if (stat(src_path, &src_stat) == -1) {
perror("stat");
exit(1);
}
if (S_ISREG(src_stat.st_mode)) {
copy_file(src_path, dst_path);
} else if (S_ISDIR(src_stat.st_mode)) {
copy_dir(src_path, dst_path);
}
}
closedir(dir);
}
```
阅读全文
相关推荐






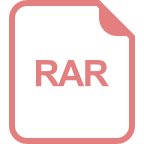






