编程在算式123 45 67 8 9=N的下划线部分填上加号(+)或减号(-),使该等式成立。要求程序运行时输出该等式。(保证数据存在满足的等式)
时间: 2024-11-20 18:34:19 浏览: 9
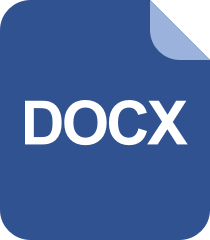
数据结构课程设计报告:在表达式“123456789=100”中左边的适当位置插入运算符“+”或“-”-以使等式成立.docx

要编写一个程序来解决这个问题,我们可以使用回溯算法或者暴力枚举的方法,尝试所有可能的组合并检查是否能找到使得等式成立的方案。因为这是一个简单的搜索问题,我们不需要担心性能问题。
以下是一个使用C语言编写的简单示例,这个程序会遍历所有可能的组合:
```c
#include <stdio.h>
#include <string.h>
int evaluate(int *arr, int n) {
int sum = arr[0];
for (int i = 1; i < n; i++) {
if (arr[i] == '+') {
sum += arr[i + 1];
} else {
sum -= arr[i + 1];
}
i++; // Skip the '+' or '-' sign
}
return sum;
}
void find_solution(int* arr, int n, char* equation, int index, bool found) {
if (index == n) {
if (evaluate(arr, n) == 9 && found) {
printf("%s\n", equation);
}
return;
}
// Try with '+'
arr[index] = '+';
find_solution(arr, n, equation, index + 2, found || (evaluate(arr, n) == 9));
// Try with '-'
arr[index] = '-';
find_solution(arr, n, equation, index + 2, found || (evaluate(arr, n) == 9));
}
int main() {
int arr[10] = {123, 45, 67, 8, 9};
char equation[20];
strcpy(equation, "123_45_67_8_9=");
find_solution(arr, 5, equation, 3, false); // Start from the first number after the numbers
return 0;
}
```
运行这段代码,如果存在满足条件的解,它将会打印出这样的等式,例如 `123 - 45 + 67 - 8 + 9 = 9` 或者其他的合法组合。
需要注意的是,这个程序并没有保证找到所有的解决方案,因为它只尝试了其中一个方向(从左到右)。如果你想要确保找到所有可能的组合,你需要调整算法以进行深度优先搜索或其他适当的搜索策略。
阅读全文
相关推荐
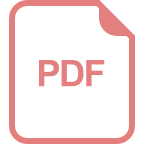
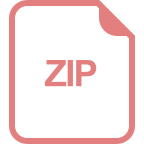

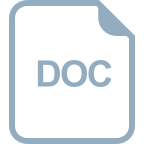
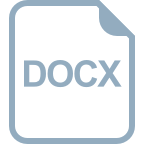
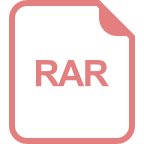
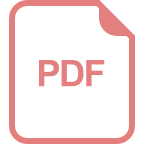
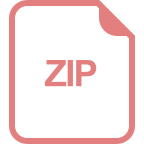
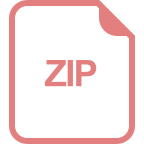
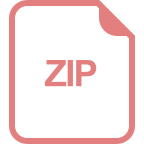
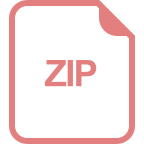
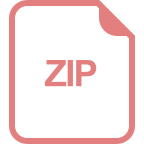
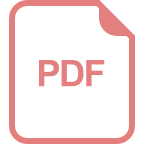
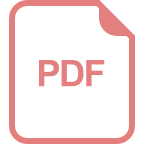
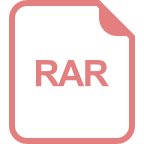