用单表输入10本书的价格,然后显示这10本书的最高价格、最低价格和平均价格Javaweb
时间: 2024-09-22 12:05:06 浏览: 68
在Java Web应用中,处理这种需求通常会涉及到前端用户界面(HTML、CSS和JavaScript)获取数据以及后端服务器(如Spring MVC或Servlets)的数据处理。这里是一个简化的步骤:
1. **前端展示**:
使用HTML表格让用户输入10本书的价格,可以使用`<form>`标签配合`<input type="number">`元素。例如:
```html
<table>
<tr>
<th>书名</th>
<th>价格</th>
</tr>
<tr>
<td><input type="text" name="bookName1"></td>
<td><input type="number" name="price1"></td>
</tr>
<!-- ...重复9次... -->
</table>
<button onclick="calculate()">计算</button>
<p id="result"></p>
```
2. **JavaScript函数**:
JavaScript负责收集数据并发送到服务器。在`calculate`函数中,计算最高价、最低价和平均价,然后更新`<p>`元素的内容:
```javascript
function calculate() {
let prices = [];
// 获取表单输入的值
// (假设每个`input`都有对应的索引)
for (let i = 1; i <= 10; i++) {
const bookPrice = document.querySelector(`[name="price${i}"]`).value;
if (bookPrice) {
prices.push(parseInt(bookPrice));
}
}
// 计算
const max = Math.max(...prices);
const min = Math.min(...prices);
const avg = prices.reduce((sum, val) => sum + val, 0) / prices.length;
// 显示结果
document.getElementById("result").textContent = `最高价:${max}, 最低价:${min}, 平均价:${avg}`;
}
```
3. **后端处理**:
后端接收前端提交的表单数据,保存到数据库或者直接返回给前端。这里假设后端API返回了数据,你可以用Ajax异步请求来获取:
```java
@GetMapping("/get-prices")
public String getBookPrices(@RequestParam int[] prices) {
// 这里对数组进行计算并返回结果
}
```
4. **页面渲染**:
在Java Web框架(如Spring MVC)中,后端计算的结果会被转发回视图模板,然后用EL表达式或Thymeleaf等技术插入到前端显示。
阅读全文
相关推荐
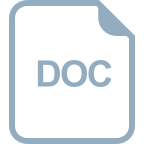
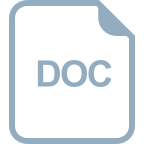
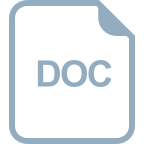
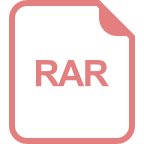
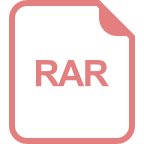
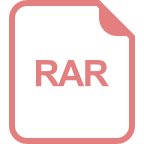
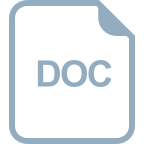
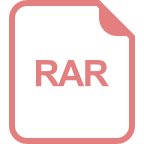
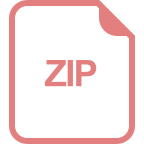
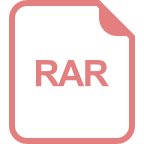
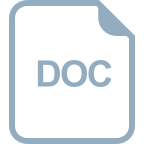
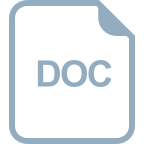