怎么使用.net framework对.net程序实现注入dll 帮我写个c++的例子
时间: 2024-02-12 07:03:47 浏览: 27
注入DLL通常需要在目标进程中创建一个远程线程,然后在远程线程中调用LoadLibrary函数加载DLL,最后调用DLL中的导出函数。以下是一个简单的C++注入DLL的示例代码:
```
#include <Windows.h>
#include <TlHelp32.h>
#include <iostream>
int main()
{
// 获取目标进程的句柄
DWORD processId = 1234; // 目标进程ID
HANDLE processHandle = OpenProcess(PROCESS_ALL_ACCESS, FALSE, processId);
if (processHandle == NULL)
{
std::cout << "Failed to open process" << std::endl;
return 1;
}
// 获取LoadLibrary函数的地址
LPVOID loadLibraryAddress = (LPVOID)GetProcAddress(GetModuleHandle("kernel32.dll"), "LoadLibraryA");
if (loadLibraryAddress == NULL)
{
std::cout << "Failed to get LoadLibrary address" << std::endl;
return 1;
}
// 分配内存
LPVOID remoteMemory = VirtualAllocEx(processHandle, NULL, MAX_PATH, MEM_COMMIT, PAGE_READWRITE);
if (remoteMemory == NULL)
{
std::cout << "Failed to allocate remote memory" << std::endl;
return 1;
}
// 写入DLL路径到目标进程中
const char* dllPath = "C:\\path\\to\\your\\dll.dll";
if (!WriteProcessMemory(processHandle, remoteMemory, dllPath, strlen(dllPath) + 1, NULL))
{
std::cout << "Failed to write DLL path to remote memory" << std::endl;
return 1;
}
// 创建远程线程并调用LoadLibrary函数
HANDLE remoteThread = CreateRemoteThread(processHandle, NULL, 0, (LPTHREAD_START_ROUTINE)loadLibraryAddress, remoteMemory, 0, NULL);
if (remoteThread == NULL)
{
std::cout << "Failed to create remote thread" << std::endl;
return 1;
}
// 等待远程线程结束
WaitForSingleObject(remoteThread, INFINITE);
// 获取导出函数地址并调用
const char* functionName = "YourExportFunction";
FARPROC functionAddress = GetProcAddress(GetModuleHandle("yourdll.dll"), functionName);
if (functionAddress == NULL)
{
std::cout << "Failed to get function address" << std::endl;
return 1;
}
// 调用导出函数
typedef int (*YourExportFunction)();
YourExportFunction yourFunction = (YourExportFunction)functionAddress;
int result = yourFunction();
std::cout << "Result: " << result << std::endl;
// 关闭句柄
CloseHandle(remoteThread);
VirtualFreeEx(processHandle, remoteMemory, 0, MEM_RELEASE);
CloseHandle(processHandle);
return 0;
}
```
在上面的示例中,我们首先使用OpenProcess函数打开目标进程句柄,然后使用GetProcAddress函数获取LoadLibrary函数的地址。接下来,我们使用VirtualAllocEx函数在目标进程中分配内存,并使用WriteProcessMemory函数将DLL路径写入目标进程中。然后,我们使用CreateRemoteThread函数创建远程线程,并在远程线程中调用LoadLibrary函数来加载DLL。最后,我们使用GetProcAddress函数获取导出函数地址,并调用导出函数。
需要注意的是,在实际的注入过程中,你还需要处理一些异常情况,例如目标进程拥有较高的权限,或者DLL文件不存在等等。此外,在使用CreateRemoteThread函数创建远程线程时,你还需要指定一个适当的起始地址,并在远程线程中调用ExitThread函数结束远程线程。
相关推荐
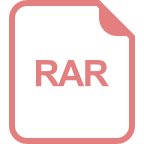













