python123列表的基本操作
时间: 2023-10-14 09:27:21 浏览: 73
Python中的列表是一种可变的序列数据类型,可以存储任意类型的元素。下面是Python列表的基本操作:
1. 创建列表
可以使用中括号 [] 来创建一个空列表,或者在中括号中添加元素来创建一个非空列表。
```python
empty_list = []
non_empty_list = [1, 2, "three"]
```
2. 访问元素
列表中的元素可以通过索引访问,索引从0开始。
```python
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # 输出:apple
```
3. 切片操作
可以使用切片操作访问列表的子集。
```python
fruits = ["apple", "banana", "cherry", "date"]
print(fruits[1:3]) # 输出:['banana', 'cherry']
```
4. 添加元素
可以使用 append() 方法在列表的末尾添加元素。
```python
fruits = ["apple", "banana", "cherry"]
fruits.append("date")
print(fruits) # 输出:['apple', 'banana', 'cherry', 'date']
```
5. 删除元素
可以使用 remove() 方法删除列表中的元素。
```python
fruits = ["apple", "banana", "cherry"]
fruits.remove("banana")
print(fruits) # 输出:['apple', 'cherry']
```
6. 修改元素
可以通过索引来修改列表中的元素。
```python
fruits = ["apple", "banana", "cherry"]
fruits[1] = "date"
print(fruits) # 输出:['apple', 'date', 'cherry']
```
7. 长度
可以使用 len() 方法来获取列表的长度。
```python
fruits = ["apple", "banana", "cherry"]
print(len(fruits)) # 输出:3
```
8. 排序
可以使用 sort() 方法对列表进行排序。
```python
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
numbers.sort()
print(numbers) # 输出:[1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
```
以上是 Python 列表的基本操作,还有很多其他的操作,可以通过 Python 的文档或搜索引擎来继续学习。
相关推荐
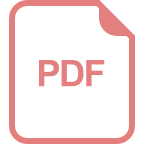
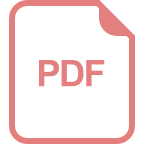
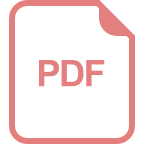
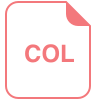
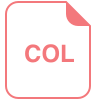
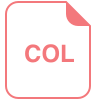
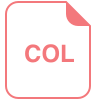
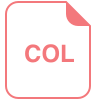









