C# WPF怎么通过弹窗来禁止用户与电脑桌面其它软件交互,只有点击弹窗上的按键才可以解除禁止
时间: 2024-06-08 14:09:35 浏览: 139
可以使用WinAPI函数来实现禁止用户与电脑桌面其他软件交互的效果,具体步骤如下:
1. 定义WinAPI函数,包括FindWindowEx、GetWindowLong、SetWindowLong、EnableWindow等。
```csharp
[DllImport("user32.dll", SetLastError = true)]
private static extern IntPtr FindWindowEx(IntPtr parentHandle, IntPtr childAfter, string className, string windowTitle);
[DllImport("user32.dll")]
private static extern int GetWindowLong(IntPtr hWnd, int nIndex);
[DllImport("user32.dll")]
private static extern int SetWindowLong(IntPtr hWnd, int nIndex, int dwNewLong);
[DllImport("user32.dll")]
private static extern bool EnableWindow(IntPtr hWnd, bool bEnable);
```
2. 获取当前应用程序的主窗口句柄,并根据需要设置其样式为无边框窗体。
```csharp
IntPtr handle = new WindowInteropHelper(Application.Current.MainWindow).Handle;
int style = GetWindowLong(handle, -20);
SetWindowLong(handle, -20, style | 0x80000 | 0x20);
```
3. 查找桌面窗口句柄,并禁用其所有子窗口。
```csharp
IntPtr desktopHandle = FindWindowEx(IntPtr.Zero, IntPtr.Zero, "Progman", null);
if (desktopHandle != IntPtr.Zero)
{
IntPtr shellHandle = FindWindowEx(desktopHandle, IntPtr.Zero, "SHELLDLL_DefView", null);
if (shellHandle != IntPtr.Zero)
{
IntPtr listViewHandle = FindWindowEx(shellHandle, IntPtr.Zero, "SysListView32", null);
if (listViewHandle != IntPtr.Zero)
{
EnableWindow(listViewHandle, false);
}
}
}
```
4. 在弹窗上添加一个按钮,当用户点击该按钮时,解除禁止。
```csharp
private void Button_Click(object sender, RoutedEventArgs e)
{
EnableWindow(listViewHandle, true);
}
```
完整代码如下:
```csharp
using System;
using System.Runtime.InteropServices;
using System.Windows;
using System.Windows.Interop;
namespace WpfApp1
{
public partial class MainWindow : Window
{
[DllImport("user32.dll", SetLastError = true)]
private static extern IntPtr FindWindowEx(IntPtr parentHandle, IntPtr childAfter, string className, string windowTitle);
[DllImport("user32.dll")]
private static extern int GetWindowLong(IntPtr hWnd, int nIndex);
[DllImport("user32.dll")]
private static extern int SetWindowLong(IntPtr hWnd, int nIndex, int dwNewLong);
[DllImport("user32.dll")]
private static extern bool EnableWindow(IntPtr hWnd, bool bEnable);
private IntPtr listViewHandle;
public MainWindow()
{
InitializeComponent();
// 获取当前应用程序的主窗口句柄
IntPtr handle = new WindowInteropHelper(Application.Current.MainWindow).Handle;
// 设置窗口样式为无边框窗体
int style = GetWindowLong(handle, -20);
SetWindowLong(handle, -20, style | 0x80000 | 0x20);
// 查找桌面窗口句柄,并禁用其所有子窗口
IntPtr desktopHandle = FindWindowEx(IntPtr.Zero, IntPtr.Zero, "Progman", null);
if (desktopHandle != IntPtr.Zero)
{
IntPtr shellHandle = FindWindowEx(desktopHandle, IntPtr.Zero, "SHELLDLL_DefView", null);
if (shellHandle != IntPtr.Zero)
{
listViewHandle = FindWindowEx(shellHandle, IntPtr.Zero, "SysListView32", null);
if (listViewHandle != IntPtr.Zero)
{
EnableWindow(listViewHandle, false);
}
}
}
}
private void Button_Click(object sender, RoutedEventArgs e)
{
// 解除禁止
EnableWindow(listViewHandle, true);
}
}
}
```
阅读全文
相关推荐
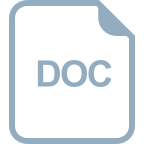
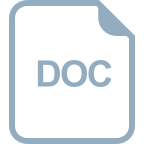
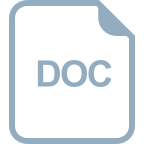


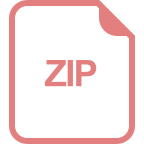

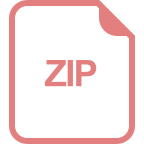
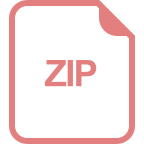
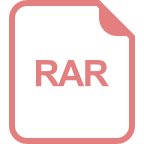
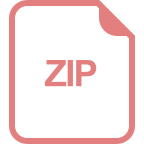
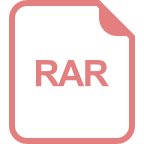
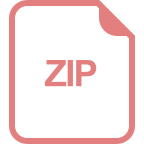
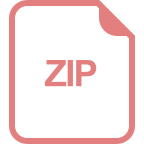
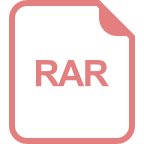
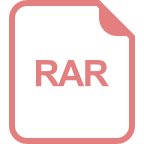
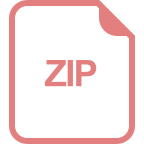