springboot excel表的导入导出
时间: 2023-07-12 13:14:17 浏览: 105
在 Spring Boot 中,可以使用 Apache POI 库来实现 Excel 表的导入和导出。以下是一个示例:
1. 导入 Excel 表
```java
@PostMapping("/import")
public String importExcel(@RequestParam("file") MultipartFile file) throws IOException {
Workbook workbook = WorkbookFactory.create(file.getInputStream());
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
String col1 = row.getCell(0).getStringCellValue();
double col2 = row.getCell(1).getNumericCellValue();
// TODO: 处理数据
}
return "导入成功";
}
```
在上述代码中,我们通过 @PostMapping 注解将一个上传文件的接口映射到 /import 路径上,通过使用 MultipartFile 类型的参数来获取上传的文件。然后,使用 WorkbookFactory 类的 create() 方法来创建 Workbook 对象,使用 getSheetAt() 方法获取 Sheet 对象,使用 for 循环遍历每一行,通过 getCell() 方法获取单元格对象,通过 getStringCellValue() 或者 getNumericCellValue() 方法获取单元格的值,最后处理数据即可。
2. 导出 Excel 表
```java
@GetMapping("/export")
public void exportExcel(HttpServletResponse response) throws IOException {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("列1");
headerRow.createCell(1).setCellValue("列2");
Row dataRow1 = sheet.createRow(1);
dataRow1.createCell(0).setCellValue("数据1");
dataRow1.createCell(1).setCellValue(123);
Row dataRow2 = sheet.createRow(2);
dataRow2.createCell(0).setCellValue("数据2");
dataRow2.createCell(1).setCellValue(456);
response.setContentType("application/vnd.ms-excel");
response.setHeader("Content-disposition", "attachment;filename=test.xlsx");
workbook.write(response.getOutputStream());
}
```
在上述代码中,我们通过 @GetMapping 注解将一个导出 Excel 表的接口映射到 /export 路径上,创建 Workbook 和 Sheet 对象,使用 createRow() 和 createCell() 方法创建行和单元格,使用 setCellValue() 方法设置单元格的值,最后将数据写入 HttpServletResponse 的输出流中即可。需要注意的是,需要设置 response 的 Content-Type 和 Content-disposition 属性来告诉浏览器下载的文件类型和文件名。
阅读全文
相关推荐
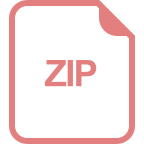
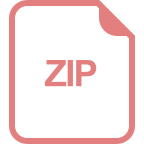
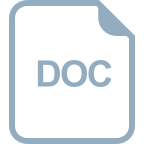



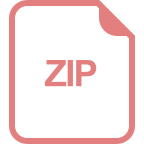
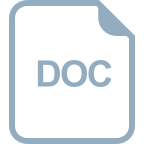








