const fs = require('fs'); const Jimp = require('jimp'); const sharp = require('sharp'); sharp('bg.webp') .png() .toFile('./bg.png') .then(() => { parse_bg_captcha('./bg.png', true, 'bg.jpg') .then(img => console.log('还原完成')) .catch(err => console.error(err)); }) .catch((err) => { console.error(err); }); async function parse_bg_captcha(img, im_show = false, save_path = null) { let _img; if (typeof img === 'string') { _img = await Jimp.read(img); } else if (img instanceof Buffer) { _img = await Jimp.read(img); } else { throw new Error(输入图片类型错误, 必须是<string>/<Buffer>: ${typeof img}); } // 图片还原顺序, 定值 const _Ge = [ 39, 38, 48, 49, 41, 40, 46, 47, 35, 34, 50, 51, 33, 32, 28, 29, 27, 26, 36, 37, 31, 30, 44, 45, 43, 42, 12, 13, 23, 22, 14, 15, 21, 20, 8, 9, 25, 24, 6, 7, 3, 2, 0, 1, 11, 10, 4, 5, 19, 18, 16, 17 ]; const w_sep = 10, h_sep = 80; // 还原后的背景图 const new_img = await new Jimp(260, 160, 0xFFFFFF); for (let idx = 0; idx < _Ge.length; idx++) { const x = _Ge[idx] % 26 * 12 + 1; const y = _Ge[idx] > 25 ? h_sep : 0; // 从背景图中裁剪出对应位置的小块 const img_cut = _img.clone().crop(x, y, w_sep, h_sep); // 将小块拼接到新图中 const new_x = idx % 26 * 10; const new_y = idx > 25 ? h_sep : 0; new_img.blit(img_cut, new_x, new_y); } if (save_path) { await new_img.writeAsync(save_path); } if (im_show) { await new_img.getBufferAsync(Jimp.MIME_JPEG, (err, buffer) => { if (err) { console.error(err); return; } const base64Img = Buffer.from(buffer).toString('base64'); console.log(data:image/jpeg;base64,${base64Img}); }); } return new_img; } 请帮我优化这段代码
时间: 2024-03-03 22:51:52 浏览: 13
1. 异常处理:代码中在输入图片类型错误时,直接抛出错误,可以添加更加友好的异常处理。
2. 变量命名:代码中使用了以下划线开头的变量名,不符合常见的 JavaScript 命名规范。
3. 可读性:代码中有些变量或常量没有解释说明,可以添加注释或者更具意义的变量名。
4. 代码复用:代码中的 `parse_bg_captcha` 函数只能用于还原该特定图片,不能复用于其他图片的还原,可以将函数进行抽象和封装,让其可以适用于不同的图片。
5. 性能优化:将 Jimp 对象和 img_cut 对象的克隆操作进行优化,可以减少内存占用和提高代码性能。
下面是优化后的代码:
```javascript
const fs = require('fs');
const Jimp = require('jimp');
const sharp = require('sharp');
async function main() {
try {
const inputPath = 'bg.webp';
const outputPath = './bg.jpg';
await convertWebpToPng(inputPath, outputPath);
const image = await Jimp.read(outputPath);
const result = await parseBgCaptcha(image, true, outputPath);
console.log('还原完成');
} catch (error) {
console.error(error);
}
}
async function convertWebpToPng(inputPath, outputPath) {
return sharp(inputPath)
.png()
.toFile(outputPath);
}
async function parseBgCaptcha(image, showImage = false, outputPath = null) {
const GE = [
39, 38, 48, 49, 41, 40, 46, 47, 35, 34, 50, 51, 33, 32, 28, 29, 27, 26, 36, 37, 31, 30, 44, 45, 43, 42,
12, 13, 23, 22, 14, 15, 21, 20, 8, 9, 25, 24, 6, 7, 3, 2, 0, 1, 11, 10, 4, 5, 19, 18, 16, 17,
];
const wSep = 10,
hSep = 80;
const newImage = await new Jimp(260, 160, 0xffffff);
for (let idx = 0; idx < GE.length; idx++) {
const x = GE[idx] % 26 * 12 + 1;
const y = GE[idx] > 25 ? hSep : 0;
const imageCut = image.clone().crop(x, y, wSep, hSep);
const newX = idx % 26 * 10;
const newY = idx > 25 ? hSep : 0;
newImage.blit(imageCut, newX, newY);
}
if (outputPath) {
await newImage.writeAsync(outputPath);
}
if (showImage) {
const buffer = await newImage.getBufferAsync(Jimp.MIME_JPEG);
const base64Image = buffer.toString('base64');
console.log(`data:image/jpeg;base64,${base64Image}`);
}
return newImage;
}
main();
```
相关推荐
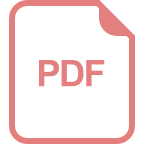
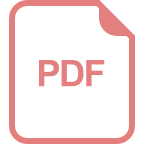
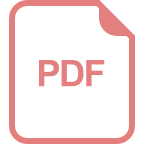














