汽车路径规划python
时间: 2023-06-29 11:05:01 浏览: 50
汽车路径规划主要是指找到从起点到终点的最短路径,同时要避免碰撞和遵守交通规则。以下是一个简单的基于Python的汽车路径规划算法示例:
首先,我们需要定义一个地图,可以使用二维数组表示。数组中的每个元素代表一个道路交叉口或路段,0表示可通过,1表示不可通过。
```python
map = [
[0, 0, 0, 0, 0],
[0, 1, 1, 0, 0],
[0, 0, 0, 1, 0],
[0, 0, 0, 1, 0],
[0, 0, 0, 0, 0]
]
```
接下来,我们需要定义起点和终点坐标。
```python
start = (0, 0)
end = (4, 4)
```
然后,我们可以使用A*算法来搜索最短路径。A*算法是一种启发式搜索算法,它可以快速找到从起点到终点的最短路径。
```python
from queue import PriorityQueue
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def astar_search(start, end, map):
frontier = PriorityQueue()
frontier.put(start, 0)
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while not frontier.empty():
current = frontier.get()
if current == end:
break
for next in get_neighbors(current, map):
new_cost = cost_so_far[current] + 1
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(end, next)
frontier.put(next, priority)
came_from[next] = current
return came_from, cost_so_far
def get_neighbors(current, map):
neighbors = []
for i in range(-1, 2):
for j in range(-1, 2):
if i == 0 and j == 0:
continue
x = current[0] + i
y = current[1] + j
if x >= 0 and x < len(map) and y >= 0 and y < len(map[0]) and map[x][y] == 0:
neighbors.append((x, y))
return neighbors
```
最后,我们可以使用得到的路径来控制汽车移动。
```python
def follow_path(path):
for i in range(len(path) - 1):
current = path[i]
next = path[i+1]
dx = next[0] - current[0]
dy = next[1] - current[1]
print("move %d,%d" % (dx, dy))
```
完整代码如下:
```python
from queue import PriorityQueue
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def astar_search(start, end, map):
frontier = PriorityQueue()
frontier.put(start, 0)
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while not frontier.empty():
current = frontier.get()
if current == end:
break
for next in get_neighbors(current, map):
new_cost = cost_so_far[current] + 1
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(end, next)
frontier.put(next, priority)
came_from[next] = current
return came_from, cost_so_far
def get_neighbors(current, map):
neighbors = []
for i in range(-1, 2):
for j in range(-1, 2):
if i == 0 and j == 0:
continue
x = current[0] + i
y = current[1] + j
if x >= 0 and x < len(map) and y >= 0 and y < len(map[0]) and map[x][y] == 0:
neighbors.append((x, y))
return neighbors
def follow_path(path):
for i in range(len(path) - 1):
current = path[i]
next = path[i+1]
dx = next[0] - current[0]
dy = next[1] - current[1]
print("move %d,%d" % (dx, dy))
map = [
[0, 0, 0, 0, 0],
[0, 1, 1, 0, 0],
[0, 0, 0, 1, 0],
[0, 0, 0, 1, 0],
[0, 0, 0, 0, 0]
]
start = (0, 0)
end = (4, 4)
came_from, cost_so_far = astar_search(start, end, map)
path = [end]
while path[-1] != start:
path.append(came_from[path[-1]])
path.reverse()
follow_path(path)
```
这是一个简单的例子,实际应用中需要考虑更多因素,例如车速、车道、交通信号等。但是这个例子可以作为一个基本的框架来进行扩展和修改。
相关推荐
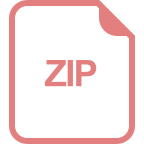
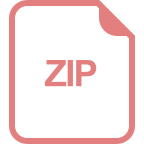
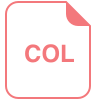
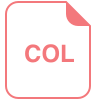
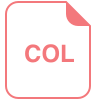
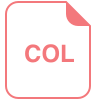
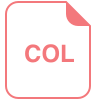









