c++面向对象程序设计:设计一个小型的图书借阅管理系统,其中涉及到两个主体:学生和图书。学生需要借阅图书。图书对象都具有相同的属性:图书条形码、图书名称、图书被借次数、是否在架;并具有相同的操作:初始化属性值、修改属性值、显示属性值。对所有图书对象抽象成一个图书类。所有的学生具有相同的属性:学号、姓名、性别、借阅册数限制、在借册数;并具有相同的操作:初始化属性值、修改属性值、显示属性值。对所有学生对象抽象成一个学生类。 学生需要借阅图书,其涉及的属性有:读者编号(即学号)、图书条形码、借出时间、归还时间;其涉及的操作有:借书、还书。给出相应代码并用main函数进行测试。
时间: 2023-07-10 12:43:03 浏览: 72
好的,下面是一个示例的 C++ 代码实现,供你参考:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <ctime>
using namespace std;
class Book {
private:
string barcode; // 图书条形码
string name; // 图书名称
int borrowed_times; // 图书被借次数
bool on_shelf; // 是否在架
public:
// 构造函数
Book(string barcode, string name, int borrowed_times = 0, bool on_shelf = true) {
this->barcode = barcode;
this->name = name;
this->borrowed_times = borrowed_times;
this->on_shelf = on_shelf;
}
// 显示属性值
void display() {
cout << "Barcode: " << barcode << endl;
cout << "Name: " << name << endl;
cout << "Borrowed times: " << borrowed_times << endl;
cout << "On shelf: " << (on_shelf ? "Yes" : "No") << endl;
}
// 修改属性值
void modify(string barcode = "", string name = "", int borrowed_times = -1, bool on_shelf = false) {
if (!barcode.empty()) this->barcode = barcode;
if (!name.empty()) this->name = name;
if (borrowed_times != -1) this->borrowed_times = borrowed_times;
if (on_shelf) this->on_shelf = on_shelf;
}
};
class Student {
private:
string student_id; // 学号
string name; // 姓名
string gender; // 性别
int borrow_limit; // 借阅册数限制
int borrowed_count; // 在借册数
public:
// 构造函数
Student(string student_id, string name, string gender, int borrow_limit = 5, int borrowed_count = 0) {
this->student_id = student_id;
this->name = name;
this->gender = gender;
this->borrow_limit = borrow_limit;
this->borrowed_count = borrowed_count;
}
// 显示属性值
void display() {
cout << "Student ID: " << student_id << endl;
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
cout << "Borrow limit: " << borrow_limit << endl;
cout << "Borrowed count: " << borrowed_count << endl;
}
// 修改属性值
void modify(string student_id = "", string name = "", string gender = "", int borrow_limit = -1, int borrowed_count = -1) {
if (!student_id.empty()) this->student_id = student_id;
if (!name.empty()) this->name = name;
if (!gender.empty()) this->gender = gender;
if (borrow_limit != -1) this->borrow_limit = borrow_limit;
if (borrowed_count != -1) this->borrowed_count = borrowed_count;
}
};
class Borrow {
private:
string student_id; // 学号
string barcode; // 图书条形码
time_t borrow_time; // 借出时间
time_t return_time; // 归还时间
public:
// 构造函数
Borrow(string student_id, string barcode) {
this->student_id = student_id;
this->barcode = barcode;
this->borrow_time = time(NULL);
this->return_time = 0;
}
// 归还图书
void return_book() {
this->return_time = time(NULL);
}
// 显示借阅信息
void display() {
cout << "Student ID: " << student_id << endl;
cout << "Barcode: " << barcode << endl;
cout << "Borrow time: " << ctime(&borrow_time);
cout << "Return time: " << (return_time ? ctime(&return_time) : "Not returned yet") << endl;
}
};
int main() {
// 初始化图书和学生
Book book1("001", "The Great Gatsby", 5, true);
Book book2("002", "To Kill a Mockingbird", 3, true);
Student student1("001", "Alice", "Female", 5, 0);
Student student2("002", "Bob", "Male", 3, 0);
// 显示图书和学生信息
cout << "Book 1:" << endl;
book1.display();
cout << endl;
cout << "Book 2:" << endl;
book2.display();
cout << endl;
cout << "Student 1:" << endl;
student1.display();
cout << endl;
cout << "Student 2:" << endl;
student2.display();
cout << endl;
// 学生借阅图书
Borrow borrow1("001", "001");
student1.modify("", "", "", 5, 1);
book1.modify("", "", 6, false);
Borrow borrow2("002", "002");
student2.modify("", "", "", 3, 1);
book2.modify("", "", 4, false);
// 显示借阅信息
cout << "Borrow 1:" << endl;
borrow1.display();
cout << endl;
cout << "Borrow 2:" << endl;
borrow2.display();
cout << endl;
// 学生归还图书
borrow1.return_book();
student1.modify("", "", "", 5, 0);
book1.modify("", "", 7, true);
borrow2.return_book();
student2.modify("", "", "", 3, 0);
book2.modify("", "", 5, true);
// 显示更新后的图书和学生信息
cout << "Book 1:" << endl;
book1.display();
cout << endl;
cout << "Book 2:" << endl;
book2.display();
cout << endl;
cout << "Student 1:" << endl;
student1.display();
cout << endl;
cout << "Student 2:" << endl;
student2.display();
cout << endl;
return 0;
}
```
在上面的代码中,我们定义了一个 `Book` 类、一个 `Student` 类和一个 `Borrow` 类,分别表示图书、学生和借阅信息。在 `Book` 类和 `Student` 类中,我们定义了相应的属性和操作,与之前的要求相同。在 `Borrow` 类中,我们定义了借阅信息的属性和操作,包括借出时间、归还时间等。在 `main` 函数中,我们初始化了两个图书和两个学生,并进行了借阅和归还操作。最后,我们显示了更新后的图书和学生信息。
阅读全文
相关推荐
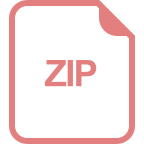
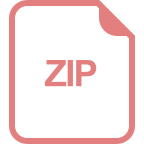
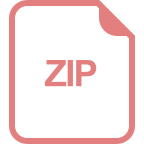
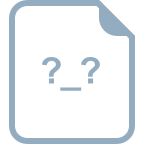
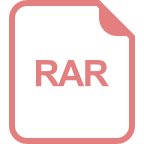
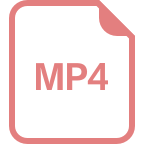
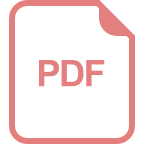