用python语言画一个动态爱心
时间: 2023-08-31 10:39:44 浏览: 75
### 回答1:
使用 Python 语言画一个动态爱心可以使用多种库,如 Matplotlib、pygame 等。具体步骤如下:
1. 安装相应库,如 Matplotlib:
```python
pip install matplotlib
```
2. 使用 Matplotlib 的函数绘制爱心形状,如:
```python
import matplotlib.pyplot as plt
import numpy as np
t = np.linspace(0, 2*np.pi, 100)
x = 16*np.sin(t)**3
y = 13*np.cos(t)-5*np.cos(2*t)-2*np.cos(3*t)-np.cos(4*t)
plt.plot(x, y)
plt.show()
```
3. 使用循环语句控制动画效果,如:
```python
import matplotlib.pyplot as plt
import numpy as np
for i in range(100):
t = np.linspace(0, 2*np.pi, 100)
x = 16*np.sin(t+i/10)**3
y = 13*np.cos(t+i/10)-5*np.cos(2*t+i/10)-2*np.cos(3*t+i/10)-np.cos(4*t+i/10)
plt.clf()
plt.plot(x, y)
plt.pause(0.1)
```
这是一种简单的实现方式,具体细节可根据需要进行修改。
### 回答2:
要用Python语言画一个动态爱心,可以通过使用turtle模块来实现。
首先,我们需要导入turtle模块:
```python
import turtle
```
然后,我们可以设置画布的背景颜色和画笔的颜色:
```python
turtle.bgcolor("black")
turtle.color("red")
```
接下来,我们可以定义一个函数来画爱心的形状:
```python
def draw_heart():
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.left(120)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.forward(224)
turtle.end_fill()
```
然后,我们可以将画笔移动到适合的位置,并开始绘制动态爱心:
```python
turtle.up()
turtle.goto(0, -100)
turtle.down()
while True:
turtle.speed(5) # 控制爱心动画速度
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
turtle.right(70)
turtle.forward(224)
turtle.left(170)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.left(140)
turtle.forward(224)
turtle.right(70)
turtle.forward(224)
turtle.end_fill()
turtle.hideturtle()
```
最后,我们可以调用`turtle.done()`来保持窗口处于打开状态,直到用户关闭窗口。
```python
turtle.done()
```
这样就可以通过Python语言绘制一个动态爱心了。希望对你有帮助!
### 回答3:
要使用Python语言画一个动态爱心,我们可以使用turtle模块来绘制图形,并通过循环和延时来实现动态效果。
首先,我们需要导入turtle模块,并设置画布的背景色和画笔的颜色。
```python
import turtle
turtle.bgcolor('black')
turtle.pensize(3)
turtle.color('red')
```
接下来,我们可以定义一个函数来画爱心图案。这个爱心图案可以由两个半圆和一个倒置的三角形组成,我们可以使用turtle的一些方法来画出它们。
```python
def draw_heart():
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.left(120)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.forward(224)
turtle.end_fill()
```
然后,我们可以使用循环和延时来动态地显示爱心图案。我们可以不断改变爱心的大小和位置,形成动画效果。
```python
while True:
turtle.speed(1)
for i in range(5):
turtle.penup()
turtle.goto(0, 0)
turtle.pendown()
draw_heart()
turtle.pensize(3)
turtle.color('red')
turtle.right(144)
turtle.hideturtle()
turtle.done()
```
最后,运行这段代码,就能够看到一个动态的爱心图案了。
综上,使用Python语言画一个动态爱心可以通过turtle模块来实现,通过循环和延时来实现动态的效果。以上代码只是一个简单的示例,你可以根据自己的需求和想法来进一步完善和扩展。
相关推荐
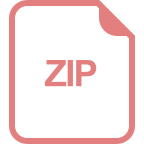
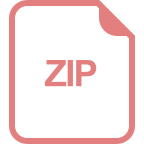
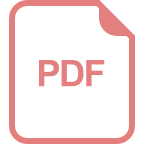














