Java中使用httputil如何发送form-data请求
时间: 2024-03-20 08:44:26 浏览: 106
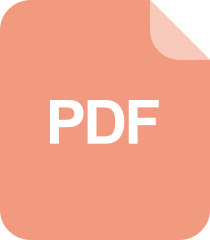
通过Java发送http请求
Java中的`httputil`没有原生的实现,但是可以使用`Apache HttpClient`库来发送`form-data`请求。以下是一个示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.HttpMultipartMode;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import java.io.File;
import java.io.IOException;
public class HttpUtil {
public static CloseableHttpResponse sendFormData(String url, String fieldName, String fileName) throws IOException {
CloseableHttpClient client = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
// 创建 multipart 请求体
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
builder.addBinaryBody(fieldName, new File(fileName), ContentType.DEFAULT_BINARY, fileName);
HttpEntity entity = builder.build();
httpPost.setEntity(entity);
// 发送请求
CloseableHttpResponse response = client.execute(httpPost);
// 处理响应
System.out.println(response.getStatusLine());
return response;
}
}
```
在调用此函数时,您需要提供目标URL,表单字段名称和文件名。例如:
```java
try {
CloseableHttpResponse response = HttpUtil.sendFormData("http://example.com/upload", "file", "/path/to/file");
// 处理响应
} catch (IOException e) {
e.printStackTrace();
}
```
请注意,您需要在代码中处理任何可能的异常,例如在发送请求或处理响应时发生的错误。
阅读全文
相关推荐

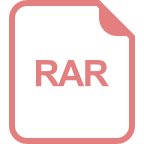
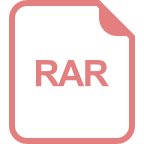
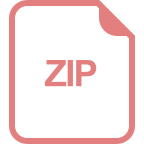
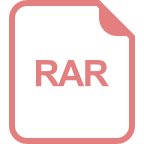
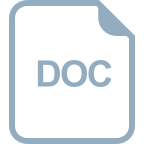
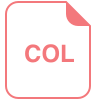
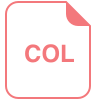







