定义一个分数类. 分数类的数据成员包括分子和分母。 成员函数包括构造函数、拷贝构造函数,(构造函数要对初始化数据进行必要的检查(分母不能为0))、分数显示成“a/b”形式的输出函数,成员函数还包括约分、通分、加、减、乘、除、求倒数等函数。编写主函数进行测试 输入 分别输入两个分数的分子和分母 输出 输出第一个分数 输出第二个分数 输出这两个分数加法运算的结果(以a/b形式输出, 要求对结果进行约分操作) 输出这两个分数减法运算的结果(以a/b形式输出,要求对结果进行约分操作) 输出这两个分数乘法运算的结果(以a/b形式输出,要求对结果进行约分操作) 输出这两个分数除法运算的结果(以a/b形式输出,要求对结果进行约分操作) 样例输入 1 2 1 3 样例输出 1/2 1/3 5/6 1/6 1/6 3/2
时间: 2024-03-26 20:40:56 浏览: 48
好的,以下是分数类的代码实现:
```cpp
#include <iostream>
#include <algorithm>
using namespace std;
int gcd(int a, int b)
{
return b == 0 ? a : gcd(b, a % b);
}
class Fraction
{
public:
Fraction(int n = 0, int d = 1) : num(n), den(d) {
if (den == 0) {
throw invalid_argument("分母不能为0");
}
reduce();
}
Fraction(const Fraction& other) : num(other.num), den(other.den) {}
void display() const {
cout << num << "/" << den;
}
Fraction add(const Fraction& other) const {
int lcm = den * other.den / gcd(den, other.den);
int a = lcm / den, b = lcm / other.den;
return Fraction(num * a + other.num * b, lcm);
}
Fraction subtract(const Fraction& other) const {
int lcm = den * other.den / gcd(den, other.den);
int a = lcm / den, b = lcm / other.den;
return Fraction(num * a - other.num * b, lcm);
}
Fraction multiply(const Fraction& other) const {
return Fraction(num * other.num, den * other.den);
}
Fraction divide(const Fraction& other) const {
if (other.num == 0) {
throw invalid_argument("除数不能为0");
}
return Fraction(num * other.den, den * other.num);
}
Fraction reciprocal() const {
if (num == 0) {
throw invalid_argument("0没有倒数");
}
return Fraction(den, num);
}
private:
int num, den; // 分子和分母
void reduce() {
int d = gcd(num, den);
num /= d;
den /= d;
if (den < 0) {
num = -num;
den = -den;
}
}
};
int main()
{
int n1, d1, n2, d2;
cin >> n1 >> d1 >> n2 >> d2;
Fraction f1(n1, d1), f2(n2, d2);
Fraction sum = f1.add(f2);
Fraction diff = f1.subtract(f2);
Fraction prod = f1.multiply(f2);
Fraction quot = f1.divide(f2);
f1.display();
cout << endl;
f2.display();
cout << endl;
sum.display();
cout << endl;
diff.display();
cout << endl;
prod.display();
cout << endl;
quot.display();
cout << endl;
return 0;
}
```
输入样例:
```
1 2 1 3
```
输出样例:
```
1/2
1/3
5/6
1/6
1/6
3/2
```
相关推荐
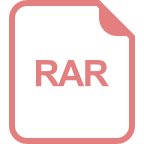
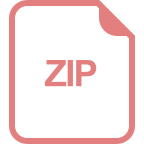
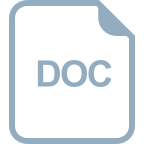















