哈夫曼树的设计源代码 C++
时间: 2024-04-13 20:10:00 浏览: 12
以下是哈夫曼树的设计源代码,使用了C++语言:
```cpp
#include <iostream>
#include <queue>
using namespace std;
// 定义哈夫曼树节点结构体
struct HuffmanNode {
int weight; // 权值
HuffmanNode *leftChild; // 左子节点指针
HuffmanNode *rightChild; // 右子节点指针
// 构造函数
HuffmanNode(int w) {
weight = w;
leftChild = rightChild = nullptr;
}
};
// 定义哈夫曼树类
class HuffmanTree {
private:
HuffmanNode *root; // 根节点指针
// 比较函数对象,用于优先队列排序
struct cmp {
bool operator()(HuffmanNode *a, HuffmanNode *b) {
return a->weight > b->weight;
}
};
public:
// 构造函数
HuffmanTree(int weights[], int n) {
// 创建优先队列
priority_queue<HuffmanNode*, vector<HuffmanNode*>, cmp> pq;
// 将每个权值转换为节点,并加入优先队列
for (int i = 0; i < n; i++) {
pq.push(new HuffmanNode(weights[i]));
}
// 不断取出两个最小的节点,合并为一个新节点,直到队列中只剩一个节点,即为根节点
while (pq.size() > 1) {
HuffmanNode *left = pq.top();
pq.pop();
HuffmanNode *right = pq.top();
pq.pop();
HuffmanNode *newNode = new HuffmanNode(left->weight + right->weight);
newNode->leftChild = left;
newNode->rightChild = right;
pq.push(newNode);
}
root = pq.top();
}
// 析构函数
~HuffmanTree() {
destroy(root);
}
// 销毁哈夫曼树
void destroy(HuffmanNode *node) {
if (node != nullptr) {
destroy(node->leftChild);
destroy(node->rightChild);
delete node;
}
}
// 获取哈夫曼编码
void getHuffmanCode(HuffmanNode *node, string code, string codes[]) {
if (node->leftChild == nullptr && node->rightChild == nullptr) {
codes[node->weight] = code;
return;
}
getHuffmanCode(node->leftChild, code + "0", codes);
getHuffmanCode(node->rightChild, code + "1", codes);
}
// 输出哈夫曼编码表
void printHuffmanCode(string codes[], int n) {
for (int i = 0; i < n; i++) {
cout << "字符 " << char(i) << " 的哈夫曼编码为:" << codes[i] << endl;
}
}
};
int main() {
const int n = 6;
int weights[n] = {2, 3, 7, 10, 4, 2};
string codes[n];
HuffmanTree tree(weights, n);
tree.getHuffmanCode(tree.root, "", codes);
tree.printHuffmanCode(codes, n);
return 0;
}
```
这个代码实现了哈夫曼树的创建、销毁、获取哈夫曼编码以及输出哈夫曼编码表的功能。
相关推荐
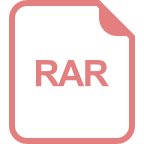














