java创建一个蔬菜类,其中包含私有属性名称、价格和重量,然 后创建方法去访问和修改的测试类
时间: 2024-05-13 13:14:51 浏览: 125
下面是一个简单的蔬菜类的Java代码,包含私有属性名称、价格和重量,以及获取和设置这些属性的方法:
```
public class Vegetable {
private String name;
private double price;
private double weight;
public Vegetable(String name, double price, double weight) {
this.name = name;
this.price = price;
this.weight = weight;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
}
```
接下来是测试类的代码,用于创建 Vegetable 对象并设置/获取属性:
```
public class TestVegetable {
public static void main(String[] args) {
Vegetable carrot = new Vegetable("Carrot", 1.99, 0.2);
System.out.println(carrot.getName() + " costs $" + carrot.getPrice() + " and weighs " + carrot.getWeight() + " kg.");
carrot.setPrice(2.49);
carrot.setWeight(0.3);
System.out.println("After update: " + carrot.getName() + " costs $" + carrot.getPrice() + " and weighs " + carrot.getWeight() + " kg.");
}
}
```
输出结果如下:
```
Carrot costs $1.99 and weighs 0.2 kg.
After update: Carrot costs $2.49 and weighs 0.3 kg.
```
在测试类中,我们首先创建一个 Vegetable 对象,然后使用 get 方法获取其属性值并打印输出。接着,我们使用 set 方法更新 Vegetable 对象的属性值,并再次打印输出。
阅读全文
相关推荐
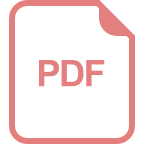
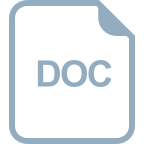






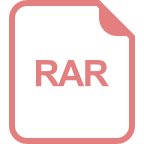
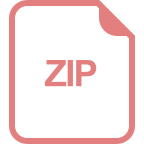
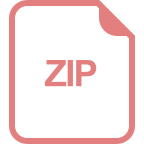
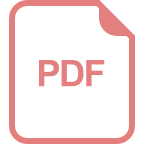
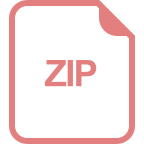
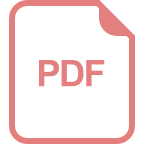
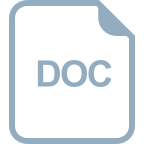
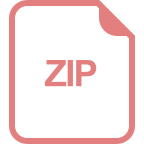
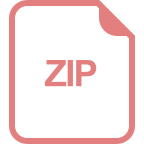
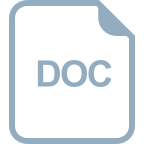