websocket心跳包c++
时间: 2023-07-04 13:09:14 浏览: 244
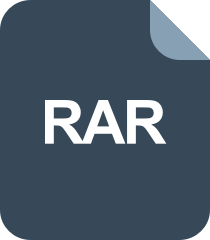
websocket 客户端 服务器 c c++实现
在C++中实现WebSocket心跳包需要使用WebSocket客户端库,比如 Boost.Beast 或者 uWebSockets。以下是使用Boost.Beast库实现WebSocket心跳包的示例代码:
```cpp
#include <boost/beast/core.hpp>
#include <boost/beast/websocket.hpp>
#include <boost/asio/ip/tcp.hpp>
#include <iostream>
using tcp = boost::asio::ip::tcp;
namespace websocket = boost::beast::websocket;
int main() {
boost::asio::io_context ioc;
tcp::resolver resolver(ioc);
auto const results = resolver.resolve("echo.websocket.org", "80");
websocket::stream<tcp::socket> ws(ioc);
boost::beast::websocket::permessage_deflate pmd;
ws.set_option(pmd);
boost::beast::websocket::stream_base::timeout opt {std::chrono::seconds(10)};
ws.set_option(opt);
boost::asio::connect(ws.next_layer(), results.begin(), results.end());
ws.handshake("echo.websocket.org", "/");
// 发送心跳包
std::string const message = "heartbeat";
boost::asio::steady_timer timer(ioc);
timer.expires_after(std::chrono::seconds(5));
timer.async_wait([&ws, &timer, &message](boost::system::error_code const& ec) {
if (!ec) {
ws.write(boost::asio::buffer(message));
timer.expires_after(std::chrono::seconds(5));
timer.async_wait([&ws, &timer, &message](boost::system::error_code const& ec) {
if (!ec) {
ws.write(boost::asio::buffer(message));
timer.expires_after(std::chrono::seconds(5));
timer.async_wait([&ws, &timer, &message](boost::system::error_code const& ec) {
if (!ec) {
ws.write(boost::asio::buffer(message));
timer.expires_after(std::chrono::seconds(5));
timer.async_wait([&ws, &timer, &message](boost::system::error_code const& ec) {
if (!ec) {
ws.write(boost::asio::buffer(message));
timer.expires_after(std::chrono::seconds(5));
timer.async_wait([&ws, &timer, &message](boost::system::error_code const& ec) {
if (!ec) {
ws.write(boost::asio::buffer(message));
}
});
}
});
}
});
}
});
}
});
ioc.run();
return 0;
}
```
上述代码中,通过Boost.Beast库创建WebSocket客户端,使用`ws.set_option()`方法设置WebSocket选项,包括心跳超时时间。然后通过`ws.handshake()`方法握手建立WebSocket连接。在发送心跳包的代码中,使用定时器定时发送心跳包并等待一定时间后再次发送心跳包。
阅读全文
相关推荐
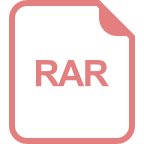
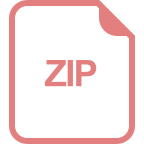














