python将dataframe写入excel指定位置
时间: 2023-05-19 17:05:53 浏览: 242
可以使用 pandas 库中的 to_excel 方法将 dataframe 写入 excel 文件,并指定写入的位置。具体代码如下:
```python
import pandas as pd
# 创建一个 dataframe
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# 将 dataframe 写入 excel 文件,并指定写入的位置
writer = pd.ExcelWriter('output.xlsx', engine='xlsxwriter')
df.to_excel(writer, sheet_name='Sheet1', startrow=1, startcol=1, index=False)
writer.save()
```
上述代码将 dataframe 写入名为 "output.xlsx" 的 excel 文件中的 "Sheet1" 工作表中,从第二行第二列开始写入,同时不写入行索引。
相关问题
python将dataframe写入excel
可以使用pandas库中的to_excel()方法将dataframe写入excel。
示例代码:
```
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df.to_excel('test.xlsx', index=False)
```
上述代码将dataframe "df" 存储为 "test.xlsx" 文件中,可以用Excel打开。
如何在python中将dataframe写入excel?
在Python中,你可以使用`pandas`库中的`to_excel()`方法将DataFrame写入Excel文件。以下是一个示例:
```python
import pandas as pd
# 创建一个DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'London', 'Paris']}
df = pd.DataFrame(data)
# 将DataFrame写入Excel文件
df.to_excel('output.xlsx', index=False)
```
在上面的示例中,我们首先创建了一个包含姓名、年龄和城市的DataFrame。然后,使用`to_excel()`方法将DataFrame写入名为`output.xlsx`的Excel文件中。`index=False`参数用于指定不将DataFrame的索引写入Excel文件。
在运行代码后,你将在当前工作目录下找到名为`output.xlsx`的Excel文件,其中包含了DataFrame的数据。
需要注意的是,你需要先安装`pandas`库,可以使用以下命令进行安装:
```
pip install pandas
```
另外,如果你需要将DataFrame写入现有的Excel文件中的特定工作表,可以使用`openpyxl`库进行操作。你可以通过指定`ExcelWriter`对象的`sheet_name`参数来实现这一点。例如:
```python
from openpyxl import load_workbook
# 加载现有的Excel文件
book = load_workbook('existing_file.xlsx')
# 创建一个ExcelWriter对象,将DataFrame写入特定工作表
writer = pd.ExcelWriter('existing_file.xlsx', engine='openpyxl')
writer.book = book
#
阅读全文
相关推荐












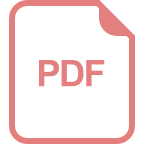
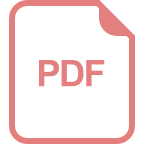