Create a Rectangle class. This class stores only the Cartesian coordinates of the four corners of the rectangle. The constructor calls a set function that accepts four sets of coordinates and verifies that each of these is in the first quadrant with no single x- or y-coordinate larger than 20.0. The set function also verifies that the supplied coordinates do, in fact, specify a rectangle. Provide member functions that calculate the length, width, perimeter and area. The length is the larger of the two dimensions. Include a predicate function square that determines whether the rectangle is a square
时间: 2024-03-23 09:38:39 浏览: 86
Sure, I can help you with that! Here is a possible implementation of the Rectangle class in Python:
```python
class Rectangle:
def __init__(self, x1, y1, x2, y2, x3, y3, x4, y4):
self.set_coordinates(x1, y1, x2, y2, x3, y3, x4, y4)
def set_coordinates(self, x1, y1, x2, y2, x3, y3, x4, y4):
# Verify that all coordinates are in the first quadrant
if x1 < 0 or y1 < 0 or x2 < 0 or y2 < 0 or x3 < 0 or y3 < 0 or x4 < 0 or y4 < 0:
raise ValueError("All coordinates must be in the first quadrant")
# Verify that no coordinate is larger than 20.0
if x1 > 20.0 or y1 > 20.0 or x2 > 20.0 or y2 > 20.0 or x3 > 20.0 or y3 > 20.0 or x4 > 20.0 or y4 > 20.0:
raise ValueError("No coordinate can be larger than 20.0")
# Verify that the coordinates specify a rectangle
if not self.is_rectangle(x1, y1, x2, y2, x3, y3, x4, y4):
raise ValueError("The coordinates do not specify a rectangle")
# Store the coordinates as a list of tuples
self.coordinates = [(x1, y1), (x2, y2), (x3, y3), (x4, y4)]
def is_rectangle(self, x1, y1, x2, y2, x3, y3, x4, y4):
# Check if the four sides have equal length and opposite sides are parallel
return self.distance(x1, y1, x2, y2) == self.distance(x3, y3, x4, y4) and \
self.distance(x2, y2, x3, y3) == self.distance(x4, y4, x1, y1) and \
abs((y2 - y1) * (y4 - y3) + (x2 - x1) * (x4 - x3)) < 1e-10
def distance(self, x1, y1, x2, y2):
# Calculate the distance between two points
return ((x2 - x1) ** 2 + (y2 - y1) ** 2) ** 0.5
def length(self):
# Calculate the length of the rectangle (longer dimension)
return max(self.distance(self.coordinates[0][0], self.coordinates[0][1],
self.coordinates[1][0], self.coordinates[1][1]),
self.distance(self.coordinates[1][0], self.coordinates[1][1],
self.coordinates[2][0], self.coordinates[2][1]))
def width(self):
# Calculate the width of the rectangle (shorter dimension)
return min(self.distance(self.coordinates[0][0], self.coordinates[0][1],
self.coordinates[1][0], self.coordinates[1][1]),
self.distance(self.coordinates[1][0], self.coordinates[1][1],
self.coordinates[2][0], self.coordinates[2][1]))
def area(self):
# Calculate the area of the rectangle
return self.length() * self.width()
def perimeter(self):
# Calculate the perimeter of the rectangle
return 2 * self.length() + 2 * self.width()
def is_square(self):
# Check if the rectangle is a square (equal length and width)
return abs(self.length() - self.width()) < 1e-10
```
Here is an example of how to use this class:
```python
# Create a rectangle with coordinates (0, 0), (0, 5), (10, 5), (10, 0)
rect = Rectangle(0, 0, 0, 5, 10, 5, 10, 0)
# Print the length, width, area, perimeter, and whether it's a square
print(rect.length()) # Output: 10.0
print(rect.width()) # Output: 5.0
print(rect.area()) # Output: 50.0
print(rect.perimeter()) # Output: 30.0
print(rect.is_square()) # Output: False
```
阅读全文
相关推荐
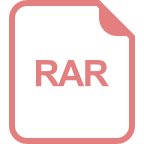
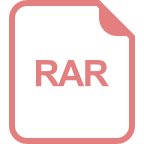
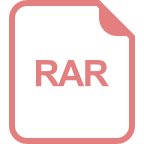
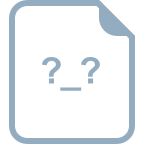
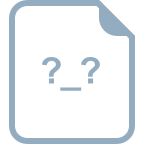
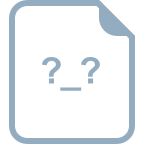
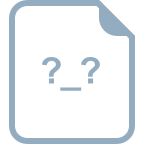
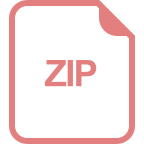
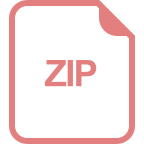