用qt写一个图片高斯滤波的程序
时间: 2023-11-26 15:05:51 浏览: 67
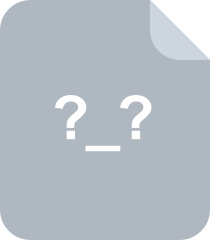
高斯滤波C程序

好的,我可以帮你回答这个问题。首先,你需要在Qt中使用OpenCV库,因为OpenCV提供了高斯滤波功能。接下来,你可以通过以下步骤来实现高斯滤波程序:
1.加载图像:使用OpenCV的imread函数来加载图像。
2.进行高斯滤波:使用OpenCV的GaussianBlur函数来进行高斯滤波。该函数需要四个参数:输入图像、输出图像、内核大小和标准差。
3.显示图像:使用Qt的QLabel和QPixmap来显示处理后的图像。
以下是一个简单的Qt代码示例,其使用OpenCV库来实现图像高斯滤波:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
#include <QMainWindow>
#include <QVBoxLayout>
#include <QGraphicsView>
#include <QGraphicsScene>
#include <QLabel>
#include <QPixmap>
using namespace std;
using namespace cv;
int main(int argc, char **argv)
{
// Load image
Mat img = imread("input.jpg");
// Apply Gaussian blur
Mat img_blur;
GaussianBlur(img, img_blur, Size(5, 5), 0);
// Convert Mat to QImage for displaying in Qt
QImage qimg((uchar*) img_blur.data, img_blur.cols, img_blur.rows, img_blur.step, QImage::Format_RGB888);
// Display processed image in a QLabel
QLabel* label = new QLabel();
label->setPixmap(QPixmap::fromImage(qimg));
label->show();
return 0;
}
```
这段代码使用imread函数加载一个名为"input.jpg"的图像,并使用GaussianBlur函数将其进行高斯滤波。然后,使用QImage将处理后的图像转换为Qt中的图像格式,并在QLabel中显示出来。
阅读全文
相关推荐
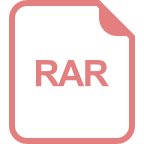
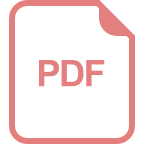


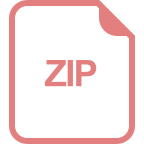
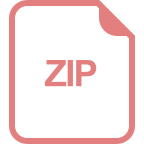
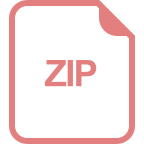
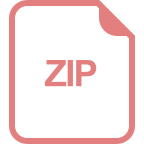
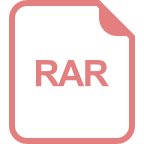
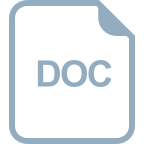

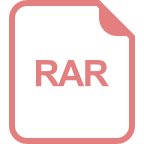
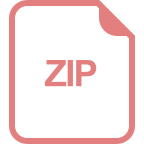
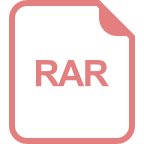
