python拼接字符串
时间: 2023-07-24 18:31:54 浏览: 85
在Python中,可以使用"+"或者"%"来拼接字符串,具体如下:
使用"+"拼接字符串:
```python
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result)
```
输出结果为:
```
Hello World
```
使用"%"拼接字符串:
```python
name = "Tom"
age = 18
result = "My name is %s, and I'm %d years old." % (name, age)
print(result)
```
输出结果为:
```
My name is Tom, and I'm 18 years old.
```
其中,"%s"表示字符串的占位符,"%d"表示整数的占位符。在字符串后面的%(name, age)中,name和age分别对应%s和%d的占位符。
相关问题
python 拼接字符串
Python中有多种方法可以拼接字符串。一种常见的方法是使用加号(+)运算符。例如,可以使用以下方式拼接两个字符串:
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
另一种方法是使用str.join()方法。它可以将一个列表内的字符串拼接成一个大的字符串。例如:
words = ["I", "love", "Python"]
result = " ".join(words)
还有一种常用的方法是使用format()方法。它可以将不同类型的数据拼接到字符串中。例如:
name = "John"
age = 25
result = "My name is {} and I am {} years old".format(name, age)
除了这些方法,还有其他一些方法可以用来拼接字符串,如使用f-string(格式化字符串)或使用字符串的乘法运算符(*)。根据不同的使用场景,可以选择最合适的方法来拼接字符串。
Python拼接字符串
Python中有多种方法可以拼接字符串,其中比较常用的方法有:
1. 使用加号(+)运算符:
```
str1 = 'hello'
str2 = 'world'
result = str1 + ' ' + str2
print(result)
```
2. 使用join方法:
```
str_list = ['hello', 'world']
result = ' '.join(str_list)
print(result)
```
3. 使用格式化字符串(f-string):
```
str1 = 'hello'
str2 = 'world'
result = f'{str1} {str2}'
print(result)
```
以上三种方法都可以用来拼接字符串,具体使用哪种方法可以根据实际情况进行选择。
阅读全文
相关推荐
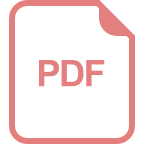
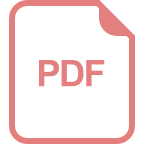
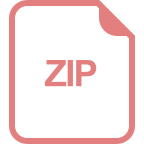













