qt qqueue入队出队的锁是同一个吗?线程里如何操作入队和出队一个消息队列,movetothread完整代码
时间: 2024-05-16 22:16:33 浏览: 126
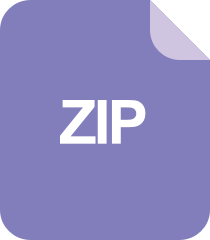
Qt中使用QQueue模拟消息队列

QQueue内部使用了线程安全的锁来保证入队和出队的操作是线程安全的。具体来说,QQueue使用了QMutex来实现锁的机制。
下面是一个入队和出队消息队列的示例代码,其中使用了QThread和QObject的movetothread方法来实现线程切换:
```cpp
// MyThread.h
#include <QThread>
#include <QQueue>
#include <QMutex>
#include <QObject>
class MyThread : public QThread
{
Q_OBJECT
public:
explicit MyThread(QObject *parent = nullptr);
virtual ~MyThread();
void addMessage(const QString& message);
signals:
void messageReceived(const QString& message);
protected:
void run() override;
private:
QQueue<QString> m_messageQueue;
QMutex m_mutex;
};
```
```cpp
// MyThread.cpp
#include "MyThread.h"
MyThread::MyThread(QObject *parent) :
QThread(parent)
{
}
MyThread::~MyThread()
{
m_mutex.lock();
m_messageQueue.clear();
m_mutex.unlock();
}
void MyThread::addMessage(const QString& message)
{
m_mutex.lock();
m_messageQueue.enqueue(message);
m_mutex.unlock();
}
void MyThread::run()
{
while (true) {
m_mutex.lock();
if (m_messageQueue.isEmpty()) {
m_mutex.unlock();
msleep(100);
} else {
QString message = m_messageQueue.dequeue();
m_mutex.unlock();
emit messageReceived(message);
}
}
}
```
在上述代码中,我们创建了一个名为MyThread的自定义线程类,其中包含一个消息队列m_messageQueue和一个QMutex锁m_mutex。addMessage方法用来在主线程中添加消息到消息队列中,run方法则在自定义线程中循环读取消息队列并逐个发送出来。
使用该类时,可以在主线程中创建一个MyThread对象并调用start方法来启动该线程,然后通过调用addMessage方法来添加消息到消息队列中。当消息队列中有消息时,MyThread类会自动将消息发送出去。示例代码如下:
```cpp
// main.cpp
#include <QCoreApplication>
#include <QDebug>
#include "MyThread.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
MyThread thread;
thread.start();
thread.moveToThread(&thread);
QObject::connect(&thread, &MyThread::messageReceived, [](const QString& message) {
qDebug() << message;
});
thread.addMessage("Hello");
thread.addMessage("World");
return a.exec();
}
```
在该示例代码中,我们创建了一个MyThread对象,并调用了start方法来启动该线程。然后,我们将该对象移动到自身所在的线程中,以便能够在自定义线程中执行run方法。最后,我们使用connect方法来将messageReceived信号连接到一个Lambda表达式中,以便能够输出接收到的消息。在调用addMessage方法时,我们向消息队列中添加了两条消息,分别为"Hello"和"World"。执行该程序后,输出结果如下:
```
"Hello"
"World"
```
可以看到,我们成功地在自定义线程中实现了一个消息队列,并且能够在主线程中向该队列中添加消息,同时在自定义线程中循环读取消息并逐个发送出去。
阅读全文
相关推荐
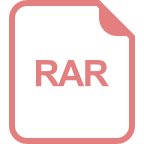
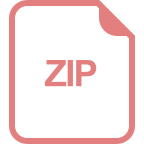















