请用多线程将这两个程序写在一起if name == 'main': app = QApplication(sys.argv) window = MyWindow() sys.stdout = window # 将标准输出重定向到 print 输出信息框中 print("print 成功") window.show() sys.exit(app.exec_()) if name == 'main': while (1): cols = ['0', '1', '2', '3', '4', '5', '6', '7'] line_names = ['VitalSigns1', 'VitalSigns2', 'VitalSigns3', 'VitalSign4', 'VitalSign5', 'VitalSign6', 'VitalSign7', 'VitalSigns8'] nowfile = time.strftime('%Y-%m-%d-%H-%M-%S', time.localtime()) if excel_name != None and over != 0: df1 = pd.read_excel(excel_name, sheet_name='Sheet1') plt.plot(df1[cols[0]], label=line_names[0]) plt.plot(df1[cols[1]], label=line_names[1]) plt.plot(df1[cols[2]], label=line_names[2]) plt.plot(df1[cols[3]], label=line_names[3]) plt.plot(df1[cols[4]], label=line_names[4]) plt.plot(df1[cols[5]], label=line_names[5]) plt.plot(df1[cols[6]], label=line_names[6]) plt.plot(df1[cols[7]], label=line_names[7]) plt.legend() plt.xlabel('NUM DATA') plt.ylabel('LIFE DATA') plt.title('Title of the plot') plt.savefig(nowfile, dpi=300) plt.show() over = 0 main()
时间: 2023-10-10 07:06:29 浏览: 108
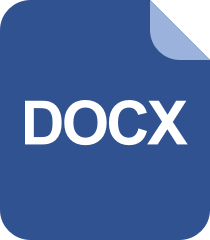
python sys.argv用法
这里提供一个简单的多线程实现,其中一个线程用来运行 GUI 程序,另一个线程用来执行数据处理和绘图操作。你需要导入 threading 模块,并创建一个新的线程来执行数据处理和绘图操作。具体实现如下:
```python
import sys
import time
import pandas as pd
import matplotlib.pyplot as plt
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit
from PyQt5.QtCore import QObject, pyqtSignal
import threading
excel_name = 'data.xlsx'
over = 1
class MyWindow(QMainWindow, QObject):
newText = pyqtSignal(str)
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(100, 100, 600, 400)
self.setWindowTitle('Print Output')
self.textEdit = QTextEdit(self)
self.textEdit.setGeometry(20, 20, 560, 360)
self.newText.connect(self.append_text)
def write(self, text):
self.newText.emit(str(text))
def flush(self):
pass
def append_text(self, text):
self.textEdit.moveCursor(6)
self.textEdit.insertPlainText(text)
def main():
global over
while True:
cols = ['0', '1', '2', '3', '4', '5', '6', '7']
line_names = ['VitalSigns1', 'VitalSigns2', 'VitalSigns3', 'VitalSign4', 'VitalSign5', 'VitalSign6', 'VitalSign7', 'VitalSigns8']
nowfile = time.strftime('%Y-%m-%d-%H-%M-%S', time.localtime())
if excel_name != None and over != 0:
df1 = pd.read_excel(excel_name, sheet_name='Sheet1')
plt.plot(df1[cols[0]], label=line_names[0])
plt.plot(df1[cols[1]], label=line_names[1])
plt.plot(df1[cols[2]], label=line_names[2])
plt.plot(df1[cols[3]], label=line_names[3])
plt.plot(df1[cols[4]], label=line_names[4])
plt.plot(df1[cols[5]], label=line_names[5])
plt.plot(df1[cols[6]], label=line_names[6])
plt.plot(df1[cols[7]], label=line_names[7])
plt.legend()
plt.xlabel('NUM DATA')
plt.ylabel('LIFE DATA')
plt.title('Title of the plot')
plt.savefig(nowfile, dpi=300)
plt.show()
over = 0
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MyWindow()
sys.stdout = window
print("print 成功")
window.show()
t = threading.Thread(target=main)
t.start()
sys.exit(app.exec_())
```
在这个实现中,新建了一个 MyWindow 类继承自 QMainWindow 和 QObject,并添加了一个 newText 信号和 append_text 槽函数用来更新 GUI 界面。MyWindow 类中重写了 write 和 flush 方法,将标准输出重定向到 GUI 界面中的 QTextEdit 控件中。
在 main 函数中,使用一个 while True 循环不断读取 excel 数据和绘图,其中 over 变量用来控制绘图操作是否执行。在主线程中运行 GUI 程序,新建一个线程来执行数据读取和绘图操作。在新线程中通过 time.sleep(1) 来控制数据处理和绘图的时间间隔。
注意,在多线程编程中,需要注意线程间的共享变量是否会发生冲突,需要使用锁来保护共享资源的访问。此处为了简化代码,没有加锁处理。在实际开发中,需要根据具体情况进行加锁处理。
阅读全文
相关推荐
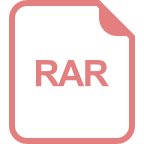
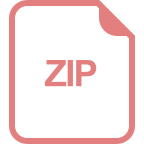
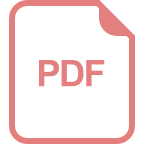
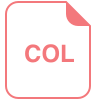
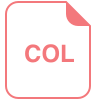
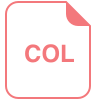
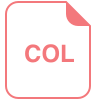
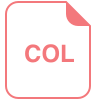
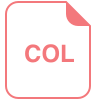




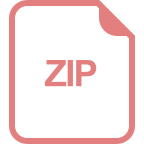