实验三shell脚本编程
时间: 2024-01-30 20:02:47 浏览: 138
好的,我们可以开始实验三的shell脚本编程。
首先,我们需要了解一些基本的shell编程语法和命令。下面是一些常用的命令:
1. echo:用于输出文本或变量的值。
2. read:用于从标准输入中读取用户输入的值。
3. if:用于条件判断。
4. for:用于循环操作。
5. while:用于循环操作。
6. case:用于多分支选择。
接下来,我们可以编写一些简单的shell脚本来练习这些命令的使用。以下是一些示例:
1. 输出Hello World
```shell
#!/bin/bash
echo "Hello World"
```
2. 读取用户输入并输出
```shell
#!/bin/bash
echo "Please enter your name:"
read name
echo "Hello, $name!"
```
3. 判断用户输入的数字是否为偶数
```shell
#!/bin/bash
echo "Please enter a number:"
read num
if [ $((num%2)) -eq 0 ]
then
echo "$num is even."
else
echo "$num is odd."
fi
```
4. 循环输出1-10的数字
```shell
#!/bin/bash
for i in {1..10}
do
echo $i
done
```
5. 循环输出当前目录下所有文件名
```shell
#!/bin/bash
for file in *
do
echo $file
done
```
6. 多分支选择
```shell
#!/bin/bash
echo "Please enter a fruit name:"
read fruit
case $fruit in
"apple")
echo "It's a kind of fruit."
;;
"potato")
echo "It's not a fruit."
;;
*)
echo "I don't know what it is."
;;
esac
```
以上是一些简单的shell脚本示例,你可以根据需要进行修改和扩展。在实验过程中,你还可以尝试使用其他的命令和语法,提高自己的shell编程能力。
阅读全文
相关推荐
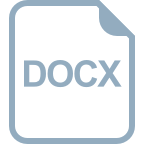
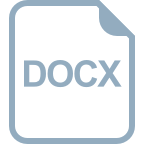
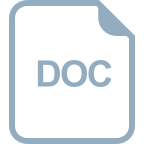
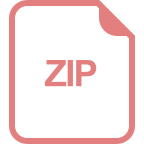
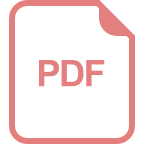
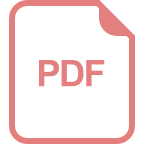
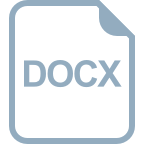
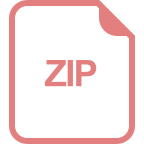
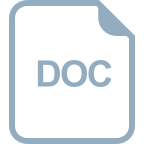
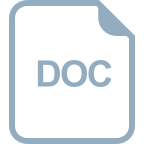
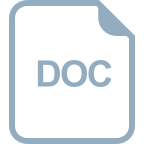
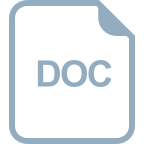
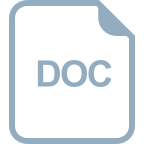
